Spring Data JPA is a powerful tool for managing persistence in any Java-based application. It helps developers easily map data from a Java application to a relational database. With Spring Data JPA, developers can quickly write data access code that is portable, maintainable, and scalable.
As the use of Spring Data JPA has become increasingly popular in recent years, many developers are eager to learn more about the technology and its applications. Interviewing for a job that requires knowledge of Spring Data JPA is a great opportunity for any developer to showcase their expertise. To help you prepare for such an interview, we have compiled a list of Spring Data JPA interview questions and answers.
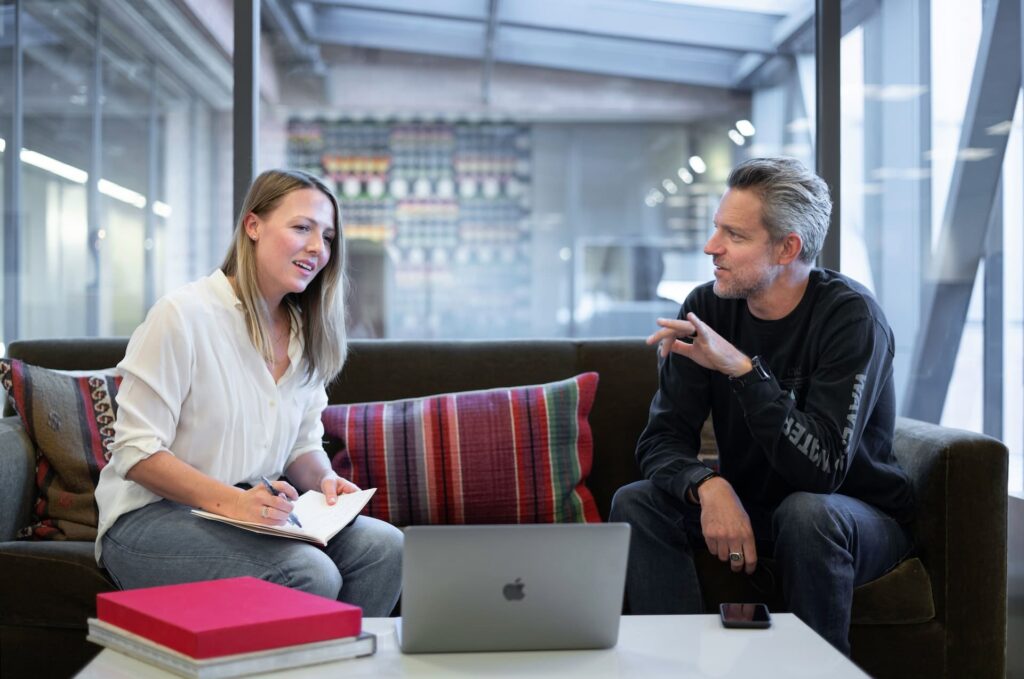
This comprehensive set of questions and answers covers all aspects of Spring Data JPA, from basic concepts to more advanced topics. Each question is accompanied by a detailed explanation of the concept being discussed, and the answers provide an in-depth understanding of the technology. Whether you are a beginner or an advanced user, these questions and answers will help you understand the fundamentals and advanced aspects of Spring Data JPA.
By going through this collection of Spring Data JPA interview questions and answers, you will be able to get a better understanding of the technology and its applications. With the right preparation, you will be able to ace your interview and demonstrate your knowledge of the technology. So, what are you waiting for? Go ahead and start brushing up on your knowledge of Spring Data JPA!
Overview of Spring Data JPA Interview Process
The Spring Data JPA interview process is focused on assessing a candidate’s knowledge of the Java Persistence API (JPA) and the Spring Data JPA framework. The process usually begins with a technical interview, during which the interviewer will ask questions about the candidate’s experience with JPA and Spring Data JPA. The candidate may be asked to explain their approach to solving data- related problems, such as mapping object- oriented classes to database tables or designing and implementing repository interfaces.
The interviewer may then ask more advanced topics, such as the advantages of using Spring Data JPA, how to create custom queries, or how to configure the persistence layer of a project. It is important for the candidate to demonstrate a good understanding of the fundamentals of the framework, as well as its features and capabilities.
The interviewer may also ask the candidate questions related to their experience with the Java Persistence API and other related technologies, such as Hibernate or EclipseLink. The candidate should be able to explain the differences between JPA and other frameworks, as well as their advantages and disadvantages.
The final stage of the interview process is typically a coding test, during which the candidate must demonstrate their skills in using JPA and Spring Data JPA to solve a given problem. This may involve writing code to implement custom repository methods, creating custom queries, or creating custom entity objects. It is important for the candidate to demonstrate that they are able to think through the problem and create a solution that meets the requirements of the task.
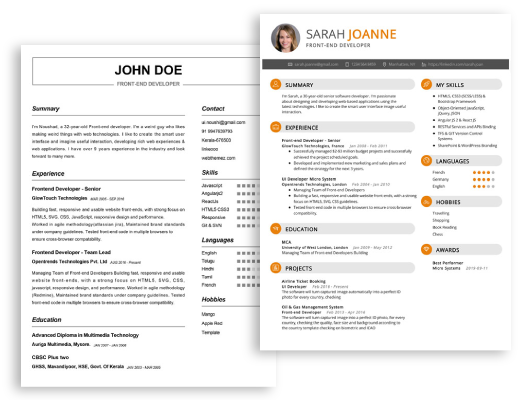
Start building your dream career today!
Create your professional resume in just 5 minutes with our easy-to-use resume builder!
Be sure to check out our resume examples, resume templates, resume formats, cover letter examples, job description, and career advice pages for more helpful tips and advice.
Top 23 Spring Data JPA Interview Questions and Answers
1. What is Spring Data JPA?
Spring Data JPA is a library that simplifies the development of Java persistence layer. It is a part of the larger Spring Data family that makes it easier to access data from different kinds of databases and data sources, such as relational databases, NoSQL databases, and cloud services. Spring Data JPA provides a specific layer of functionality to help developers work with databases and the Java Persistence API (JPA). Spring Data JPA allows developers to easily map Java objects to database tables, and to create hand-written database queries by using standard JPA annotations and query methods. This reduces the amount of code needed to access data, and makes it easier for developers to keep their code up to date with database schema changes.
2. What is the benefit of using Spring Data JPA?
The primary benefit of using Spring Data JPA is that it reduces the amount of boilerplate code required for database access. It also makes it easier for developers to keep their code up to date with database schema changes, since the annotations and query methods used to access data can be updated to reflect the new schema. Additionally, Spring Data JPA provides a range of tools to help developers debug and optimize their database access code, such as query execution logging, performance monitoring, and query plan analysis.
3. What is ORM (Object-Relational Mapping)?
Object-Relational Mapping (ORM) is the process of mapping objects in a program to objects in a database. ORM allows developers to access data in a relational database using their programming language of choice, such as Java or C#. ORM libraries, such as JPA or Hibernate, can provide a layer of abstraction between the database and the application, which makes it easier for developers to access data without worrying about the underlying database structure.
4. What is the difference between Spring Data JPA and Hibernate?
Spring Data JPA is a library that simplifies the development of Java persistence layer, while Hibernate is an ORM framework that allows developers to access relational databases using their programming language of choice. Both technologies can be used to access data in a relational database, but they serve different purposes. Spring Data JPA provides a layer of abstraction between the application and the database, simplifying database access and making it easier to keep the code up to date with database schema changes. Hibernate, on the other hand, provides a richer set of features for manipulating and querying data, such as caching and lazy loading.
5. What is the difference between Spring Data JPA and Spring Data?
Spring Data JPA is a library that simplifies the development of Java persistence layer, while Spring Data is a larger family of libraries for accessing data from different kinds of databases and data sources, such as relational databases, NoSQL databases, and cloud services. Both technologies can be used to access data in a database, but they serve different purposes. Spring Data JPA provides a layer of abstraction between the application and the database, simplifying database access and making it easier to keep the code up to date with database schema changes. Spring Data, on the other hand, provides a range of tools and libraries for accessing data from different kinds of databases and data sources.
6. How do you create a custom query using Spring Data JPA?
Spring Data JPA allows developers to create custom queries using the @Query annotation. The @Query annotation can be used to define a native SQL query, or a JPQL query. For example, a query can be defined as follows:
@Query(“SELECT c FROM Customer c WHERE c.name LIKE :name”)
List findByName(String name);
This query will return a list of customers whose name matches the given name.
7. What are the advantages of using Spring Data JPA?
The primary advantage of using Spring Data JPA is that it reduces the amount of boilerplate code required for database access. It also makes it easier for developers to keep their code up to date with database schema changes, since the annotations and query methods used to access data can be updated to reflect the new schema. Additionally, Spring Data JPA provides a range of tools to help developers debug and optimize their database access code, such as query execution logging, performance monitoring, and query plan analysis.
8. What are the different types of query methods that can be used with Spring Data JPA?
The most common type of query method used with Spring Data JPA is the named query. Named queries are defined using the @NamedQuery annotation and can be used to define a query in a single location and refer to it from multiple locations in the application. Other types of query methods include derived queries, which use method names to derive queries from the method name, and native queries, which allow developers to define a native SQL query using the @Query annotation.
9. What is the difference between a repository and a service in Spring Data JPA?
A repository is a component in Spring Data JPA that is used to access data from the database. It provides a layer of abstraction between the application and the database, simplifying database access and making it easier to keep the code up to date with database schema changes. A service, on the other hand, is a component in Spring Data JPA that provides a higher level of abstraction than a repository. It is used to define business logic and perform operations that span multiple repositories.
10. How do you set the fetch size in Spring Data JPA?
The fetch size in Spring Data JPA can be set using the @QueryHints annotation. The @QueryHints annotation allows developers to specify a map of query hints that will be used to customize the query when it is executed. To set the fetch size, use the @QueryHints annotation and set the value of the FETCH_SIZE hint to the desired value. For example, the following code snippet can be used to set the fetch size to 100:
@QueryHints({@QueryHint(name=”org.hibernate.fetchSize”, value=”100″)})
List findByName(String name);
11. What is the difference between @Query and @NamedQuery in Spring Data JPA?
The @Query annotation is used to define a native SQL query, or a JPQL query, whereas the @NamedQuery annotation is used to define a named query. Named queries are defined in a single location and can be referred to from multiple locations in the application. They can also be used to optimize the performance of queries by allowing the query to be pre-parsed and cached.
12. What are the different types of query parameters supported by Spring Data JPA?
Spring Data JPA supports the following types of query parameters: positional parameters, named parameters, and named native query parameters. Positional parameters use the question mark (?) character to mark the parameter in the query, and are numbered in the order they appear in the query. Named parameters use the colon (:) character to mark the parameter in the query and are specified using a name. Named native query parameters are only supported for native queries and use the colon (:) character to mark the parameter in the query.
13. What is the difference between @Modifying and @Transactional in Spring Data JPA?
The @Modifying annotation is used to indicate that the query being executed should be executed as a write operation. This allows the query to be executed as part of a transaction. The @Transactional annotation is used to mark a method as being part of a transaction. It allows the query to be executed within a transaction, and ensures that any changes made to the database are rolled back if an error occurs.
14. What is the difference between CrudRepository and JpaRepository in Spring Data JPA?
The CrudRepository interface provides basic CRUD operations for entities, such as save, find, delete, and count. The JpaRepository interface provides additional operations for entities, such as find by specific fields, sorting, and pagination. Additionally, the JpaRepository interface provides support for the Java Persistence API (JPA).
15. What is the difference between findOne() and findById() in Spring Data JPA?
The findOne() method is used to find an entity by its ID. It will return a Optional object containing the entity, or an empty Optional if the entity is not found. The findById() method is used to find an entity by its ID. It will return a null if the entity is not found, or the entity if it is found.
16. What is the difference between save() and save And Flush() in Spring Data JPA?
The save() method is used to save an entity to the database. It will not flush any changes to the database, so any changes made to the entity will not be persisted until the session is flushed. The saveAndFlush() method is used to save an entity to the database and then flush the changes to the database.
17. How do you set the isolation level in Spring Data JPA?
The isolation level in Spring Data JPA can be set using the @Transactional annotation. The @Transactional annotation allows developers to specify the isolation level as a parameter of the annotation.
18. What is the difference between JPA and Spring Data JPA?
JPA (Java Persistence API) is a specification for accessing, persisting, and managing data between Java objects/classes and a relational database. Spring Data JPA is a library/framework that adds an extra layer of abstraction on top of JPA and helps to reduce the boilerplate code and complexity of JPA. It provides an advanced set of features such as native queries, improved transaction management, and simplified data access layer development. It also provides enhanced support for repositories, making it easier to create data access objects (DAOs) for persistent objects.
19. How do you configure Spring Data JPA?
To configure Spring Data JPA, you need to first add the necessary dependencies to the project. Spring Data JPA requires the Spring Data Commons, Spring Data JPA, and the JPA implementation to be present in the project. Next, you need to create a configuration class and annotate it with @EnableJpaRepositories. This annotation tells Spring Data to create a JPA repository instance for each entity. Finally, you need to define the JPA configuration, including the JPA vendor adapter, data source, and the packages to be scanned for entities.
20. What is a JPA repository in Spring Data JPA?
A JPA repository is a data access object (DAO) that provides an interface for interacting with a JPA-managed database. It uses the JPA specification to provide an object-oriented API for performing common data access operations, such as creating, reading, updating, and deleting entities. A JPA repository can be created with Spring Data JPA by annotating an interface with the @Repository annotation. The interface should extend the JpaRepository interface, which provides the necessary methods for interacting with the database.
21. What are the main annotations used in Spring Data JPA?
The main annotations used in Spring Data JPA are @Entity, @Id, @Table, @Column, @Transient, @ManyToOne, @OneToMany, @ManyToMany, @Embeddable, and @EmbeddedId. These annotations are used to define the entities, the primary keys, the tables, the columns, the relationships, and the embeddable objects. Additionally, there are annotations that can be used to customize the queries, such as @Query and @QueryParam.
22. How does Spring Data JPA simplify the development process?
Spring Data JPA simplifies the development process by providing an abstraction layer to the underlying database. This abstraction layer makes it easier to write code that interacts with the data source in an object-oriented manner. Additionally, it provides support for query languages, such as JPQL and Criteria API, which makes it easier to write database queries. Finally, it provides support for transactions, making it easier to manage data access in a multi-threaded or distributed environment.
23. What is Query DSL?
Query DSL (Domain-Specific Language) is a language that is used to create type-safe queries for Spring Data JPA repositories. It is an alternative to writing query methods in SQL, as it provides an easier way to write queries. Query DSL is composed of a set of keywords that can be used to create type-safe queries. These keywords can be used to define criteria, sorting, pagination, and projection of data.
Tips on Preparing for a Spring Data JPA Interview
- Research the company’s technology stack and best practices for Spring Data JPA.
- Refresh your knowledge of the features and capabilities of the Spring Data JPA Framework.
- Practice working session questions related to Spring Data JPA.
- Prepare a portfolio of data projects or applications you have created using Spring Data JPA.
- Review the interview questions that might be asked and have answers prepared.
- Understand the fundamentals of relational databases and the Object Relational Mapping (ORM) concept.
- Understand the JPA query language and be able to explain how to build queries.
- Learn the differences between HQL and JPQL and practice coding in both.
- Familiarize yourself with the different types of transactions and their implications in a Spring Data JPA application.
- Understand the different levels of caching and their implications for performance.
- Know how to configure the Spring Data JPA application for optimal performance.
- Be able to explain how the data is stored in a Spring Data JPA application.
- Prepare questions that you can ask the interviewer about their experience with Spring Data JPA.
- Practice writing code in a short amount of time.
- Learn about the latest features of the Spring Data JPA framework.
Conclusion
In conclusion, Spring Data JPA is an essential technology for Java developers who need to manage and access data in a Java- based environment. This technology is powerful, yet relatively easy to learn, and is great for increasing productivity in both large and small applications. An understanding of the fundamentals, as well as the ability to answer the most commonly asked Spring Data JPA interview questions, is key to having a successful job interview. With the knowledge gained from this article, you should be well on your way to having a successful job interview.