PHP Object Oriented Programming (OOPs) is an important concept for web developers who utilize the PHP language. OOPs is a programming paradigm that increases the flexibility and scalability of applications. It is a programming language that allows developers to define the data type of a variable, define the relationships between different objects, and create and manipulate objects.
Interviewers often ask questions about PHP OOPs in interviews to test the knowledge of a candidate. These questions range from the basics of OOPs to more advanced concepts such as inheritance, encapsulation, and abstract classes.
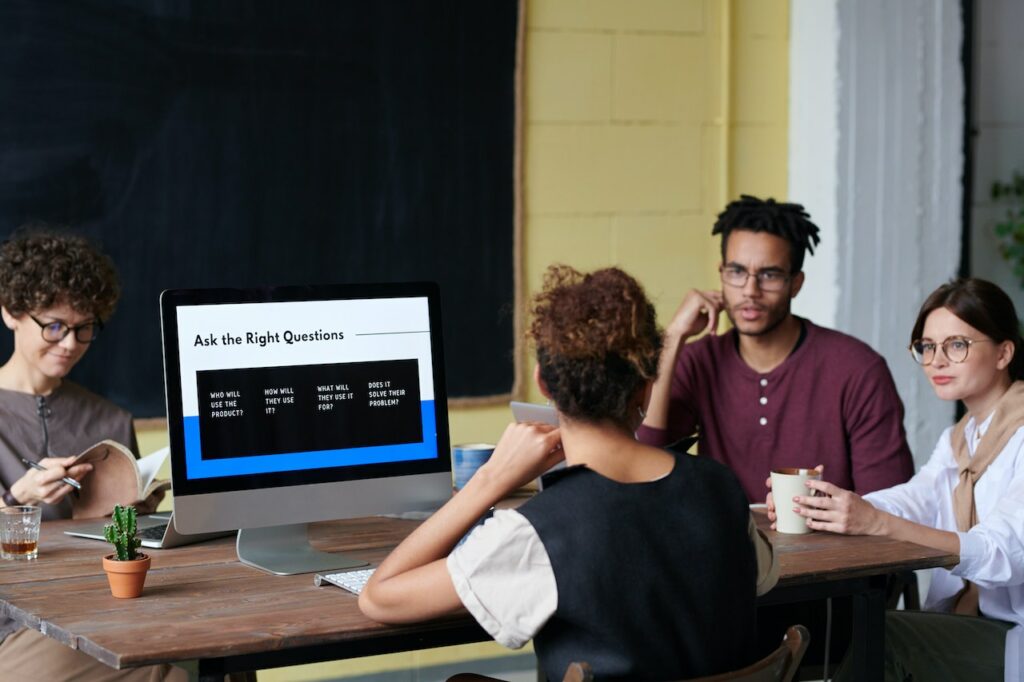
This blog post provides answers to some of the common OOPs interview questions in the context of PHP. It covers the basics such as the definition of OOPs, the principles of OOPs, and the basic syntax of the language. It also includes more complex topics such as inheritance, abstraction, and polymorphism. Each answer is accompanied by an example to help clarify the concepts.
By reading this blog, you will gain a better understanding of the fundamentals of PHP OOPs and be able to answer the most common interview questions about the topic. This blog post is a must-read for any web developer who is looking to brush up on their OOPs knowledge and prepare for a job interview.
Overview of PHP Oops Interview Process
The interview process for PHP OOPs typically begins with a pre- screening phone call or a video call. During this call, the interviewer will ask questions about the candidate’s technical skills, experience, and knowledge of PHP OOPs. They will also ask questions about their background, education, and career goals.
If the candidate passes the pre- screening, they will usually be asked to come into the office for an in- person interview. During this meeting, the interviewer will ask more detailed questions about the candidate’s experience, knowledge, and skills related to PHP OOPs.
The interviewer will also likely ask questions to gauge the candidate’s problem- solving and critical thinking skills, as well as any questions related to the specific project they are interviewing for. The interviewer may also present the candidate with a few coding challenges related to PHP OOPs to assess the candidate’s ability to understand and work with the language.
Once the in- person interview is complete, the candidate may be asked to participate in a second round of interviews. During these, the interviewer may ask more technical questions and may also give the candidate an opportunity to present a project or code they’ve written.
At the end of the process, the interviewer will often ask the candidate for references or for a portfolio of past projects or coding samples. After the interviewer reviews these, they will typically make their decision about whether to hire the candidate.
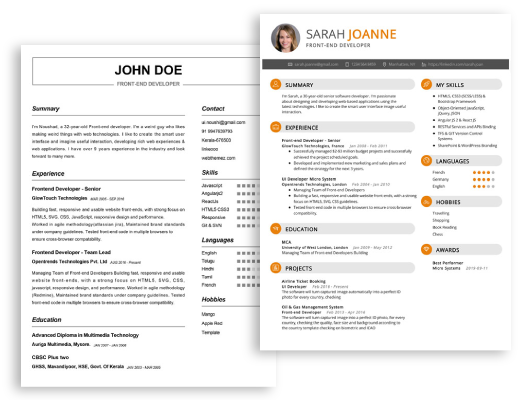
Start building your dream career today!
Create your professional resume in just 5 minutes with our easy-to-use resume builder!
Be sure to check out our resume examples, resume templates, resume formats, cover letter examples, job description, and career advice pages for more helpful tips and advice.
Top 23 PHP Oops Interview Questions and Answers
1. What is OOPs?
Object Orientated Programming (OOP) is a programming language model in which programs are organized around objects rather than “actions” and data rather than logic. It provides a way of modularizing programs by creating partitions of responsibility which makes it easier to understand and maintain. OOP also makes it easier to create reusable code and develop programs that are efficient and easy to use.
2. What is a class?
A class is a blueprint or template for creating objects. It is the most fundamental element of object-oriented programming. A class contains variables (known as properties) and methods (known as functions). By using classes we can create objects which can interact with each other by using their properties and methods.
3. What is an object?
An object is an instance of a class which has its own state and behavior. It is a self-contained entity that consists of methods and properties to make up its behavior. Objects are created from classes by using the new keyword.
4. What is inheritance?
Inheritance is a way of providing a relationship between classes. It allows one class to inherit the properties and methods of another class. This allows for code reusability and makes it easier to extend the functionality of a program.
5. What is an interface?
An interface is a way of providing a contract between two classes. It is an abstract class that defines the methods that must be implemented by any class that implements the interface. It is used to provide a common set of methods that all classes must implement.
6. What is Polymorphisim?
Polymorphisim is the ability of an object to take on different forms. It allows an object to behave differently depending on the context in which it is used. Polymorphisim is one of the four pillars of Object Oriented Programming and allows for code reuse.
7. What are abstract classes?
Abstract classes are classes that cannot be instantiated. They are used to provide a common set of methods and properties that all classes must implement. Abstract classes are used to provide a base for other classes to extend and build upon.
8. What are abstract methods?
Abstract methods are methods that are declared in an abstract class, but not implemented. Abstract methods must be implemented in any class that extends the abstract class. Abstract methods help to ensure that all classes that extend the abstract class implement the same methods.
9. What are traits?
Traits are a way of providing code reusability in PHP. They allow a class to use methods and properties from another class without having to extend it. Traits can be used to provide common methods and properties that can be used by multiple classes.
10. What is the difference between a class and an interface?
The main difference between a class and an interface is that a class can be instantiated and an interface cannot. A class is a blueprint or template for creating objects, while an interface is an abstract class which defines the methods that must be implemented by any class that implements the interface.
11. What is the difference between an abstract class and a trait?
The main difference between an abstract class and a trait is that an abstract class is a class that cannot be instantiated, while a trait is a way of providing code reusability in PHP. An abstract class is used to provide a common set of methods and properties that all classes must implement, while a trait allows a class to use methods and properties from another class without having to extend it.
12. What is the difference between a method and a function?
The main difference between a method and a function is that a method is a function that is declared within a class, while a function is a standalone piece of code that can be called from anywhere. A method can access the properties and methods of the class it is declared in, while a function cannot.
13. What is the difference between public, protected and private keywords?
The public, protected and private keywords are used to specify the scope of a class’ members. Public members are accessible to anyone, protected members are only accessible to the class itself and its child classes, and private members are only accessible to the class itself.
14. What is the use of the final keyword?
The final keyword is used to prevent a class or method from being overridden. It is used to ensure that the code remains unchanged and is not modified by any class or method that extends or implements the class or method.
15. What is the use of “::” operator?
The “::” operator is used to access static methods and properties of a class. It allows us to access methods and properties without having to create an instance of the class.
16. What is Object-Oriented Programming (OOP)?
Object-oriented programming (OOP) is a programming paradigm that relies on objects and classes to create programs and applications. It is a type of programming language that focuses on objects rather than functions and data. Objects are collections of data, and they can interact with one another. Each object has its own properties and methods which can be accessed and manipulated by other objects. OOP helps to create an intuitive, modular, and efficient code base that is easy to maintain and extend.
17. What are the main principles of OOP?
The main principles of OOP include encapsulation, abstraction, inheritance, and polymorphism. Encapsulation means that the code and data associated with an object are kept together, which allows for better data security and code reuse. Abstraction is the process of hiding implementation details from the user. Inheritance allows for the reuse of code, by allowing objects to inherit methods and properties from parent objects. Finally, polymorphism is the ability of an object to act differently depending on the context.
18. What is the difference between a class and an object?
A class is a template or blueprint which is used to create objects. It defines the characteristics, behavior, and properties of an object. An object is an instance of a class, meaning that it is a concrete representation of a particular class. An object contains its own data, methods, properties, and variables.
19. What are the advantages of using object-oriented programming?
Object-oriented programming has many advantages, including improved code reusability, better data security, and easier code maintenance. Reusing code can save time, as well as make the code more efficient and secure. Additionally, OOP helps to create a more modular and intuitive code base, which makes it easier to maintain and extend.
20. What is encapsulation in OOP?
Encapsulation is a principle of OOP which focuses on keeping the code and data associated with an object together. This makes it easier to secure the data, as well as reuse code. It also helps to create a more intuitive and modular code base which is easier to maintain and extend.
21. What is inheritance in OOP?
Inheritance is a principle of OOP which allows objects to inherit methods and properties from parent objects. This helps to create a more efficient code base, as well as make it easier to maintain and extend.
22. What is the difference between composition and inheritance?
The main difference between composition and inheritance is that composition allows classes to reuse code by combining existing objects, while inheritance allows objects to reuse code by inheriting methods and properties from parent objects. Both methods help to create a more efficient code base and make it easier to maintain and extend.
23. What is the difference between public, protected, and private access modifiers?
Public access modifiers allow objects to access the data or methods of a class from any location. Protected access modifiers allow objects to access the data or methods of a class from within the same class or from a subclass. Private access modifiers restrict access to the data or methods of a class to within the same class. These access modifiers are used to control access to data and methods of a class, making it easier to maintain and extend the code.
Tips on Preparing for a PHP Oops Interview
- Brush up on the basics of Object- Oriented Programming (OOP) and review PHP documentation on OOP principles and coding.
- Get familiar with the most popular PHP frameworks that utilize OOP principles, such as Laravel and Symfony.
- Research and practice coding in Object- Oriented style and refactor existing code into OOP.
- Be familiar with the main concepts of OOP such as classes, objects, properties, methods, inheritance, abstraction, polymorphism, and encapsulation.
- Have examples of projects you’ve worked on that included OOP code and be able to explain your coding process.
- Understand how to implement design patterns in OOP PHP, such as Singleton and Factory patterns.
- Be able to explain the differences between procedural and object- oriented coding and the advantages of each.
- Have an understanding of the differences between PHP 5 and 7 versions, in particular how they affect OOP coding.
- Be able to explain the differences between classes, interfaces, and traits.
- Have a working knowledge of different databases and their interactions with OOP coding.
- Understand how to debug and use error handling with OOP coding.
- Demonstrate an understanding of the various PHP OOP libraries.
- Have an understanding of refactoring and optimization techniques for OOP coding.
- Know the differences between objects and variables and the scope of each.
- Be able to explain the security implications of OOP and the best practices for coding securely.
Conclusion
PHP OOPs Interview Questions and Answers are designed to help individuals gain a better understanding of how PHP OOPs works and how to answer questions related to it during an interview. By understanding the key concepts of OOPs and being able to answer related questions in an interview, you can make sure that you stand out from other applicants and get the job you want. Learning about PHP OOPs and the related questions can give you a better idea of what you need to know to land the job you have been looking for.