RxJS is a JavaScript library that provides reactive programming support which enables developers to integrate asynchronous data streams into their applications. It is a popular library among developers due to its wide range of features and flexibility. RxJS is used to create highly scalable and maintainable applications.
RxJS is an incredibly powerful tool and understanding it can be difficult. In order to get the most of out of RxJS, it is important to learn the fundamentals and understand how to use it properly. Interviewing for a job that requires RxJS knowledge can be intimidating, so it is important to have a good understanding of the basics.
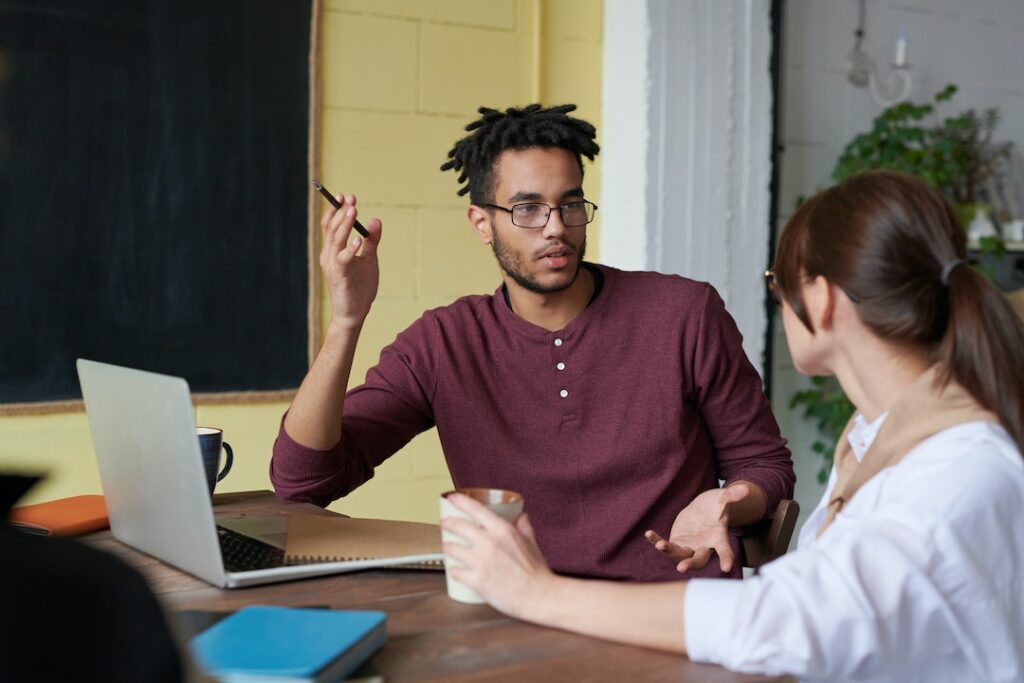
In this blog, we will provide you with the most commonly asked RxJS interview questions and answers. We will go over the basic concepts, such as the core principles of reactive programming, the RxJS operators, and the differences between RxJS and other libraries. We will also discuss more advanced topics such as best practices, debugging, and optimization. After reading this blog, you should have a comprehensive understanding of RxJS and be better prepared for a job interview.
Overview of RXJS Interview Process
The RXJS interview process is a process that enables employers to assess a candidate’s knowledge and skills around the reactive programming library for JavaScript called RXJS. This process typically involves a combination of written tests and interviews, depending on the complexity of the job and the amount of technical knowledge required.
The first step of the process is typically a written test that serves as a screening tool. In this case, employers will ask candidates to answer a series of questions related to the library. This can include questions related to the basics of the library such as syntax and common operations, or it could include more advanced topics such as observables and streams. These questions can be used to determine the candidate’s level of knowledge, and to identify areas of improvement.
The next step is usually an interview. This can take the form of a one- on- one or panel interview, and will involve questions related to the candidate’s experience, skills, and understanding of the library. Employers may ask candidates to explain how they implemented a certain feature, why they chose a particular approach, or how they debugged a problem with the library. Employers may also ask candidates to demonstrate their understanding of certain concepts by providing examples.
Lastly, employers may also ask candidates to complete a coding challenge. This could involve asking candidates to build an application or solve a specific problem using the library. This can be a great way to assess a candidate’s technical abilities and problem- solving skills.
Overall, employers use the RXJS interview process to determine if a candidate has the necessary knowledge and experience to work with the library and contribute to their team. It’s important for candidates to prepare for the process by doing research on the library and understanding the topics that could be covered in the written tests and interviews.
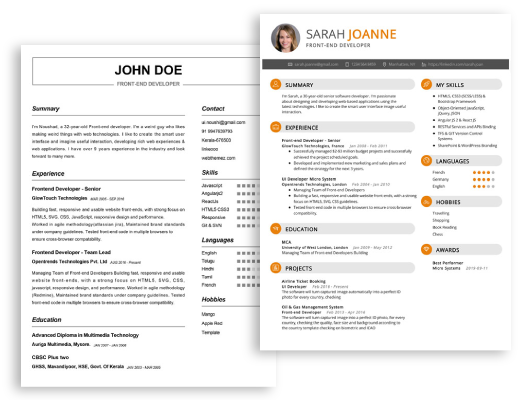
Start building your dream career today!
Create your professional resume in just 5 minutes with our easy-to-use resume builder!
Be sure to check out our resume examples, resume templates, resume formats, cover letter examples, job description, and career advice pages for more helpful tips and advice.
Top 20 RXJS Interview Questions and Answers
1. What is RXJS?
RxJS (Reactive Extensions Library for JavaScript) is a library for reactive programming using observables that makes it easier to compose asynchronous or callback-based code. It provides one core type, the Observable, satellite types (Observer, Schedulers, Subjects) and operators inspired by Array#extras (map, filter, reduce, every, etc.) to allow handling asynchronous events as collections.
2. What are observables in RXJS?
Observables are objects in RxJS that emit data over time. When observables are subscribed to, they become data producers. Observables are the foundation of the RxJS library, and they provide a powerful way to work with asynchronous data streams.
3. What are the benefits of using RXJS?
RxJS allows you to create reactive data streams that can be composed together, allowing for efficient and elegant code. With RxJS, you can create streams of data and manipulate them as needed, as well as easily create complex data flows. Additionally, RxJS provides a way to handle errors and handle asynchronous tasks in a clean and concise way.
4. What is a Subscription in RXJS?
A subscription is an object that represents a disposable resource, usually the execution of a Observable. Subscriptions are created when an observer subscribes to a Observable. Subscription provides a way to unsubscribe from a Observable. Subscriptions are important for controlling the number of resources that an application uses.
5. What is a Subject in RxJS?
Subjects are a special type of Observable that allows values to be multicasted to many Observers. Subjects are like EventEmitters: they maintain a registry of many listeners. They keep track of Observers that subscribe to them, and they can send values to all of the registered Observers.
6. What is a Scheduler in RxJS?
A Scheduler is an object used to schedule code to be executed in the future. It can be used to schedule code to be run at a certain time, or at a certain interval. Schedulers provide a way to control the timing of execution of code.
7. What are the different types of Schedulers in RxJS?
RxJS provides a number of different types of Schedulers for controlling the execution of code. These include: ImmediateScheduler, asapScheduler, queueScheduler, animationFrameScheduler, as well as VirtualTimeScheduler.
8. What is an Operator in RxJS?
Operators are pure functions that enable a wide range of functional capabilities including transformation, filtering, joining, and error handling. Operators can be used with Observables to transform, filter, or combine data streams before they are consumed by Observers.
9. What is a Cold Observable in RxJS?
A Cold Observable is an Observable that does not begin to emit values until it is subscribed to. Cold Observables are often used for data streams that are expensive to produce, or that are generated from an external source.
10. What is a Hot Observable in RxJS?
A Hot Observable is an Observable that begins to emit values as soon as it is created, even if there are no Observers subscribed to it. Hot Observables are often used for data streams that are generated from within the application, like user input events.
11. What is an AsyncSubject in RxJS?
An AsyncSubject is a type of Subject that only emits the last value of the Observable sequence. It is used for cases where the last value of a data stream is the most important one.
12. What is a BehaviorSubject in RxJS?
A BehaviorSubject is a type of Subject that emits the most recently emitted value from the source Observable as soon as it is subscribed to. It is useful for preserving the current state of an Observable sequence, as well as for providing the initial value for an Observer on subscription.
13. What is a ReplaySubject in RxJS?
A ReplaySubject is a type of Subject that emits a specified number of previously emitted values from the source Observable to Observers when they subscribe. It is useful for buffering data from an Observable sequence, as well as for refiring events when a new Observer subscribes.
14. What is an Observable Query in RxJS?
An Observable Query is a mechanism for creating a data stream from a set of queries that are provided by the user. It is similar to a traditional database query, but with the added benefit of being able to provide the results in real-time, as the data is updated.
15. What is a Multicasted Observable in RxJS?
A Multicasted Observable is an Observable that can be subscribed to by multiple Observers. It allows a single source Observable to be shared among multiple Observers, and any data that is emitted from the source will be received by all of the Observers.
16. What is an Error Handling Operator in RxJS?
Error Handling Operators are operators that are designed to handle errors that occur in an Observable sequence. They provide a way to catch errors and carry out specific actions like logging errors, retrying operations, or routing to an alternative Observable sequence.
17. What is the switchMap operator in RxJS?
The switchMap operator is a form of the map operator that allows for a given Observable to switch to a different Observable when the data from the source Observable changes. This is useful for situations where the source Observable changes rapidly, as it ensures that the data from the source Observable is always used.
18. What is the debounce operator in RxJS?
The debounce operator is an operator that waits for a specified amount of time before emitting a value from the source Observable. It is useful for scenarios where a user is typing in a text box and you only want to process the input once they have finished typing.
19. What is the takeUntil operator in RxJS?
The takeUntil operator is an operator that takes values from the source Observable until a given condition is met. It is useful for scenarios where you want to stop processing data from the source Observable after a certain condition is met.
20. What is the mergeMap operator in RxJS?
The mergeMap operator is a form of the map operator that allows for multiple Observables to be merged together into one Observable. It is useful for scenarios where you have data from several different sources that need to be merged together into a single stream of data.
Tips on Preparing for a RXJS Interview
- Review the core concepts of RxJS, such as observables, operators, and subscriptions.
- Understand the basic syntax of RxJS, including the use of the pipe() operator.
- Understand the purpose and differences between subjects, observables and observers.
- Learn the basics of libraries and frameworks associated with RxJS such as Angular and Redux.
- Read about common use cases for RxJS and be prepared to discuss them.
- Understand and be able to explain the asynchronous nature of RxJS.
- Become familiar with the most commonly used RxJS operators and be able to explain how they work.
- Be able to explain the concept of lazy evaluation and how it relates to RxJS.
- Be able to identify and explain data transformations that are possible with RxJS.
- Prepare examples of projects or tasks you’ve completed that utilized RxJS.
- Be prepared to explain how you debugged asynchronous code with RxJS.
- Understand how error handling works in RxJS and be able to discuss best practices.
- Be able to discuss the differences between RxJS and other reactive programming libraries.
- Understand the purpose of schedulers and be able to explain when they should be used.
- Familiarize yourself with the latest features and changes to RxJS.
Conclusion
Overall, RXJS is a powerful library for managing asynchronous data streams. With its simple API and wide range of functions, it makes it easy to create complex reactive applications. These RXJS interview questions and answers provide a great starting point for anyone looking to dive into RxJS and gain a better understanding of the library. By understanding the fundamentals, developers can quickly learn how to use RSXJS and build powerful applications.