An interview is the only way to get hired for a job and to stand out among the competition. But it can be a daunting task, especially when it comes to the tricky questions that are asked during the interview. Proper preparation is key, and one of the best ways to prepare is by going through the list of pointers interview questions and answers.
Pointers are a concept used in computer programming, specifically in the C and C++ programming languages. It is a special type of variable that can store the address of another variable, making it a powerful tool for managing memory. Knowing how pointers work and how to use them is essential for any aspiring programmer.
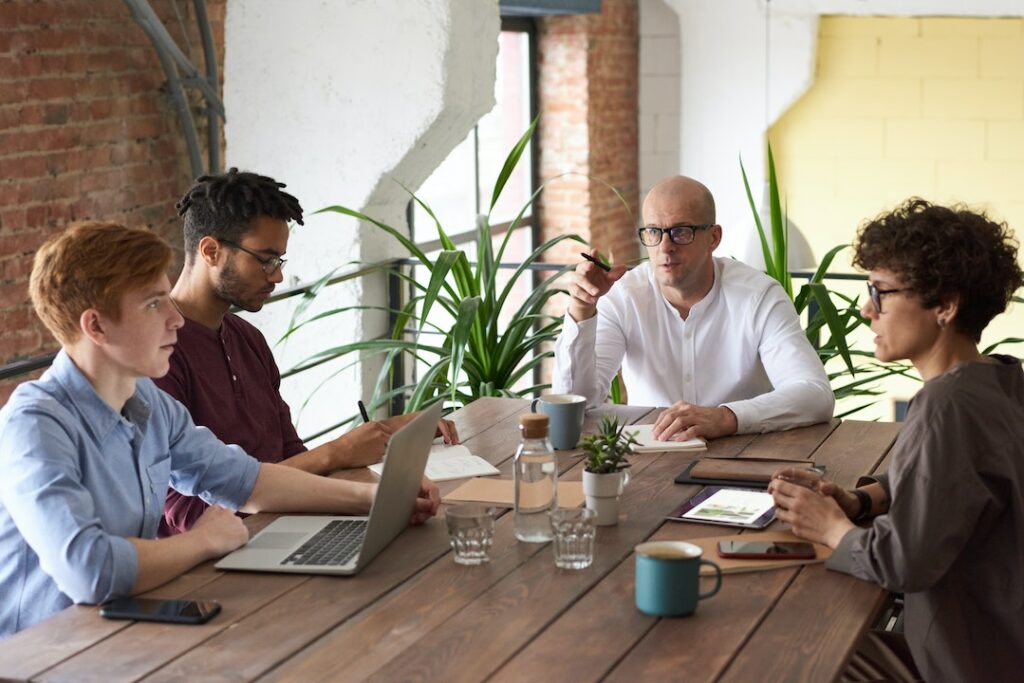
With that in mind, here we have compiled a list of pointers interview questions and answers to help you prepare for your next interview. This list includes some of the most common questions asked about pointers and their practical use. It also includes some pointers questions in the form of code snippets and quizzes, so you can practice implementing these concepts.
The questions in this list include topics such as the definition of pointers, uses of pointers, pointer arithmetic, memory allocation, pointer manipulation, and more. We have also added some example scenarios to help you understand how to use pointers in programming.
In order to ace your next interview, remember to familiarize yourself with the concept of pointers and test your knowledge with the helpful questions and answers provided here. With adequate preparation, you can be sure that you will be able to answer any pointers questions asked during the interview.
Overview of Pointers Interview Process
The pointers interview process typically begins with a preliminary phone screen. During the phone screen, the hiring manager or recruiter will ask a series of questions to gauge the applicant’s technical aptitude and experience with coding languages like C++, as well as their understanding of pointers. Following the phone screen, applicants may be asked to complete a coding challenge that includes working with pointers.
The next stage of the pointers interview process is typically a one- on- one technical interview. During this interview, the interviewer will ask questions about understanding and implementing pointers in programming, as well as questions about algorithms, data structures, and memory management. The interviewer may also ask applicants to write code to demonstrate their knowledge of pointers.
The last step of the pointers interview process is often a technical panel interview in which the applicant answers questions from multiple members of the technical team. Panel interviews can be quite challenging, as they require applicants to quickly and accurately answer questions from multiple sources at once. The panel will typically ask questions that are more complex than the ones asked in the previous stages and test the applicant’s ability to think critically and come up with solutions on the spot.
Overall, the pointers interview process can be a lengthy and challenging process. However, if applicants come prepared with a thorough understanding of pointers and coding languages like C++, they can make it through the process with success.
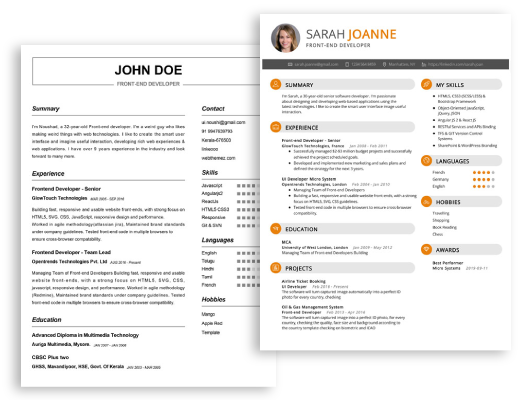
Start building your dream career today!
Create your professional resume in just 5 minutes with our easy-to-use resume builder!
Be sure to check out our resume examples, resume templates, resume formats, cover letter examples, job description, and career advice pages for more helpful tips and advice.
Top 20 Pointers Interview Questions and Answers
1. Explain what a pointer is?
A pointer is a variable in programming that stores the address of another variable. It points to a specific memory address of a value that is stored in memory. A pointer can be used to refer to the contents of the memory address, such as to assign or modify a value stored there. Pointers are commonly used to efficiently pass parameters to functions, for dynamic memory allocation, for data structures such as linked lists and trees, and to create alias names for existing variables.
2. What is the difference between a pointer and a reference?
A pointer is a variable that holds the memory address of another variable, while a reference is an alias name for an existing variable. The main difference is that a pointer can point to a different memory address, while a reference always refers to the same memory address. Also, a pointer can be re-assigned to point to a different memory address, while a reference can’t.
3. What is the difference between normal variables and pointers?
Normal variables are allocated memory on the stack, while pointers are allocated memory on the heap. Normal variables can hold any type of data, while pointers can only hold the address of another variable. Normal variables can be assigned a value directly, while pointers have to be assigned the address of an existing variable. Finally, normal variables are not affected by reference or pointer operations, while pointers can be affected.
4. What are the benefits of using pointers?
Using pointers can allow for more efficient memory management. By using pointers instead of normal variables, developers can allocate memory on the heap, which is much larger than the stack and is not limited by the size of the program. Pointers can also be used to pass parameters to functions much more efficiently than normal variables. Finally, pointers can be used to create efficient data structures, such as linked lists and trees, which can help improve the performance of applications.
5. What is pointer arithmetic?
Pointer arithmetic is the process of adding or subtracting from a pointer’s value. This is done by either incrementing or decrementing the pointer’s value. This can be used to traverse an array or to access specific elements in memory. Additionally, pointer arithmetic can be used to find the distance between two pointers.
6. What is the ‘null pointer’?
A null pointer is a pointer that points to nothing. It is usually set to the memory address 0, and is often used to indicate the end of a list or that a pointer is not pointing to any valid memory address.
7. What is a void pointer?
A void pointer is a pointer that can point to any type of data. It is commonly used when a function needs to return a pointer to an unknown type of data, or when a pointer needs to be passed as a parameter to a function that accepts any type of pointer.
8. What is the difference between a pointer and an array?
An array is a collection of variables of the same type that is allocated in contiguous memory. A pointer, on the other hand, is a variable that holds the memory address of another variable. Arrays can be used to store multiple values, while pointers can only be used to refer to one value. Additionally, an array can be indexed, while pointers have to be dereferenced to access the value that they point to.
9. What is dynamic memory allocation?
Dynamic memory allocation is the process of allocating memory at runtime. It allows a program to allocate memory when it is needed, rather than allocating all of the memory at compile time. Dynamic memory allocation is commonly used to improve the performance of applications, as it can allow for more efficient memory management.
10. What is the difference between malloc() and calloc()?
Malloc() is used to allocate a block of memory, while calloc() is used to allocate a block of memory and initialize it to zero. Malloc() requires the program to manually initialize the block of memory, while calloc() automatically initializes the block of memory. Additionally, malloc() requires the program to manually deallocate the block of memory, while calloc() automatically deallocates the block of memory.
11. What is the difference between malloc() and new?
Malloc() is a C library function that is used to allocate memory at runtime, while new is an operator that is used to allocate memory at runtime. Both malloc() and new can be used to allocate memory, but new is more commonly used in C++ due to its support for various features such as type safety, exception handling, and initialization.
12. What is dereferencing a pointer?
Dereferencing a pointer is the process of accessing the value of a memory address that the pointer is pointing to. This is done by using the dereference operator, which is the asterisk (*). For example, if a pointer is pointing to the memory address 0x100, then dereferencing the pointer will access the value stored at that memory address.
13. What is an object pointer?
An object pointer is a pointer that points to an object, which is an instance of a class. Object pointers can be used to refer to the members and functions of a class, allowing for the manipulation of objects. Additionally, object pointers can be used to create linked lists and other data structures that can help improve the performance of applications.
14. What is a ‘this’ pointer?
A ‘this’ pointer is a pointer that points to the current instance of a class. It is passed as an implicit parameter to all member functions of a class, allowing the function to access the members and functions of the class.
15. What is a dangling pointer?
A dangling pointer is a pointer that points to a memory location that has been deallocated or is invalid. Dangling pointers can lead to segmentation faults and can cause a program to crash.
16. How can dangling pointers be avoided?
Dangling pointers can be avoided by checking if a pointer is still pointing to a valid memory address before using it. Additionally, it is important to make sure that any memory that is allocated is properly deallocated when it is no longer needed.
17. What is a wild pointer?
A wild pointer is a pointer that has not been initialized. It is pointing to an unknown memory address and can be dangerous as it can cause segmentation faults and program crashes.
18. How can wild pointers be avoided?
Wild pointers can be avoided by making sure that all pointers are properly initialized before they are used. Additionally, it is important to make sure that variables are always assigned valid values and that they are not left uninitialized.
19. What is a smart pointer?
A smart pointer is a type of pointer that provides additional features such as memory management and exception safety. It automatically deallocates memory when it is no longer needed, and can be used to replace regular pointers.
20. What is a weak pointer?
A weak pointer is a pointer that points to an object, but does not increase its reference count. This means that when the object is deleted, the weak pointer does not prevent it from being deleted. Weak pointers can be used to prevent memory leaks and can help improve the performance of applications.
Tips on Preparing for a Pointers Interview
- Familiarize yourself with basic pointer concepts such as memory allocation, address spaces, and pointer arithmetic.
- Review the syntax and usage of pointers in the language you’ll be using in the interview.
- Practice coding problems that involve pointers and dynamic data structures.
- Have an understanding of the different types of pointers and the various operations that can be performed on them.
- Brush up on the fundamentals of the language you’ll be using for the interview.
- Understand the differences between passing pointers and passing by value.
- Understand the differences between pointer variables and references.
- Be able to explain pointer- based algorithms and data structures.
- Have a good understanding of memory management and memory leaks.
- Be able to explain the differences between pointers and arrays.
- Understand object- oriented programming concepts and how they relate to pointers.
- Be able to explain the differences between local and global pointers.
- Have an understanding of pointer optimization techniques.
- Practice writing programs with pointers.
- Brush up on the difference between pointers and references.
Conclusion
In conclusion, when it comes to interviewing for a position that involves using pointers, it is important to be prepared with the right set of questions and answers. Having a good understanding of C & C++ pointers and their uses can help you ace the interview. Knowing the different types of pointers, their scope, and their applications is essential, as well as being able to explain the differences between them and how they can be used effectively. With practice, you can become an expert in pointer interview questions and answers, and secure your dream job.