Inheritance is one of the most important concepts in object-oriented programming and is used to enable code reuse. It is a powerful concept that allows developers to create complex and robust systems. Understanding inheritance and its principles is essential for any programmer or software engineer. This blog post covers the most common inheritance interview questions and answers.
Inheritance is the process by which one class acquires the properties and characteristics of another class. This process allows for code reuse, which in turn makes code more efficient and resilient when compared to starting from scratch. It also allows for the ability to easily extend or modify existing code. With the use of inheritance, developers can write code that is more maintainable and robust.
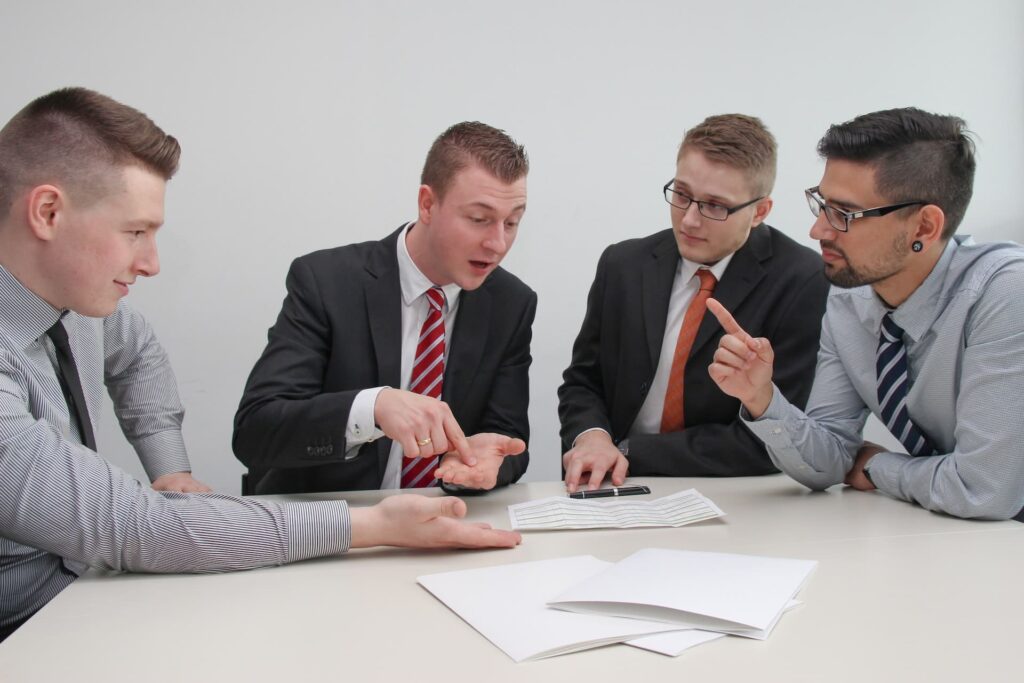
The inheritance interview questions and answers covered in this blog post are designed to illustrate the basics of inheritance and its principles. They are aimed at developers who are looking to gain a better understanding of the concept and its many uses. The questions will cover topics such as the types of inheritance, the differences between inheritance and composition, and the advantages and disadvantages of using inheritance.
By understanding the basics of inheritance, developers can create more efficient and maintainable code. This post will provide a comprehensive overview of the most common inheritance interview questions and answers that will help developers gain a better understanding of the concept.
Overview of Inheritance Interview Process
The inheritance interview process is a method used by attorneys, financial professionals, and other experts to help families explore their estate and inheritance planning. The process begins with an initial consultation, where the estate planer will ask questions about the family members and the assets in the estate. During the consultation, the estate planner will discuss the relative strengths and weaknesses of the estate, and suggest strategies to maximize the estate’s value.
The next step in the inheritance interview process is to develop a plan of action. In this phase, the estate planner will work with the family to create an estate plan that meets their goals and objectives. The plan will include an inventory of the assets, an analysis of the family’s tax situation, and an assessment of the legal implications of any decisions. The estate planner will also provide advice on how to best manage the estate and ensure that the family’s wishes are carried out in the event of death.
The next step in the process is to review the estate plan with the family. During this phase, the estate planner will review the plan with the family, answer any questions, and modify the plan if necessary. This review process is critical in ensuring that the estate plan meets the family’s goals and objectives.
The last step in the process is to implement the estate plan. This may involve setting up trusts or other vehicles to transfer assets to beneficiaries, or distributing assets to heirs directly. Once the estate plan is in place, the estate planner will provide ongoing management and advice to ensure that the plan is being followed.
Overall, the inheritance interview process is a comprehensive approach to estate planning. It helps families to understand the assets in their estate, evaluate the tax implication of their decisions, and develop a plan that meets their goals and objectives. The process also ensures that the family’s wishes are followed and the estate plan is implemented properly.
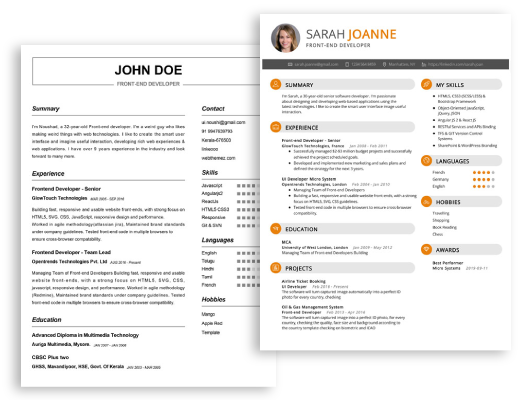
Start building your dream career today!
Create your professional resume in just 5 minutes with our easy-to-use resume builder!
Be sure to check out our resume examples, resume templates, resume formats, cover letter examples, job description, and career advice pages for more helpful tips and advice.
Top 20 Inheritance Interview Questions and Answers
1. What is inheritance?
Inheritance is a way of organizing code by allowing one class (the child class) to take on the properties and methods of another class (the parent class). This allows for code to be reused and makes it easier to expand and maintain existing code. Additionally, it allows new classes to be created from existing classes, which is a great way to reduce time and effort when writing a new program.
2. What is a derived class?
A derived class (also known as a child class or subclass) is a class that is created from another class (often referred to as the parent or base class). The derived class takes on all the properties and methods of the parent class, but can also have its own properties and methods that are unique to the derived class.
3. What is polymorphism?
Polymorphism is a way of allowing an object to take on multiple forms. In object-oriented programming, this can mean allowing an object to take on multiple methods or behaviors depending on the context. For example, an Animal object might have different methods for eat(), sleep(), and run(), depending on what type of animal it is.
4. What is an abstract class?
An abstract class is a class that is not meant to be instantiated, but serves as a template from which other classes can be derived. It is a way of organizing code to allow for common properties and methods to be shared by all objects that are derived from the abstract class.
5. What is the difference between single inheritance and multiple inheritance?
Single inheritance is when a class inherits from one parent class, while multiple inheritance is when a class inherits from multiple parent classes. Single inheritance is simpler and easier to maintain than multiple inheritance, which can become complex quickly.
6. What are the benefits of inheritance?
Inheritance is a great way to save time and effort when writing a program, as it allows for code to be reused and expanded upon. It also helps to keep code organized and maintainable, as code can be split into different classes that are related to each other. Additionally, it allows for new classes to be created from existing classes, which helps with code reuse and scalability.
7. What is the purpose of the super keyword?
The super keyword is used to access the properties and methods of the parent class from within the child class. It is useful for accessing methods in the parent class that have been overridden (redefined) in the child class.
8. What is the difference between composition and inheritance?
Composition is when an object contains other objects, while inheritance is when a class inherits from another class. Composition is useful for creating complex objects and is often used to create objects that have a hierarchical structure. Inheritance, on the other hand, is useful for code reuse and is often used to create new classes that are related to existing classes.
9. What is method overriding?
Method overriding is when a method in a child class has the same name and signature as a method in a parent class. When this happens, the method in the child class will take precedence and be used instead of the method in the parent class. This is useful for modifying or extending the behavior of existing methods.
10. What is an abstract method?
An abstract method is a method that is declared but not implemented in a class. It is typically used as a placeholder for a method that will be implemented in a derived class. Abstract methods are often used to enforce a particular behavior in a class hierarchy.
11. What is the difference between a virtual method and an abstract method?
A virtual method is a method that can be overridden in a derived class, while an abstract method is a method that is declared but not implemented in a class. Virtual methods are used to modify the behavior of a class, while abstract methods are used to enforce a particular behavior in a class hierarchy.
12. What is a protected member in a class?
A protected member is a member of a class that can only be accessed from within the class and its derived classes. It is a way of encapsulating data and methods, as it prevents outside classes from accessing the protected member.
13. What is the difference between an interface and an abstract class?
An interface is a way of defining a contract between classes, while an abstract class is a class that is not meant to be instantiated, but serves as a template from which other classes can be derived. An interface defines a set of methods that must be implemented in a class, while an abstract class can contain both implemented and abstract methods.
14. What is the purpose of a constructor in a class?
A constructor is a special method that is used to create an instance of a class. It is a way of setting up the initial state of an object and is typically used to initialize member variables or perform other setup tasks.
15. What is the purpose of a destructor in a class?
A destructor is a special method that is used to clean up an instance of a class before it is destroyed. It is a way of releasing any resources that the object may have acquired so that they can be reused by other objects.
16. What are the types of inheritance supported by C#?
C# supports single inheritance and multiple inheritance. Single inheritance is when a class inherits from one parent class, while multiple inheritance is when a class inherits from multiple parent classes.
17. How do you prevent a class from being inherited?
A class can be prevented from being inherited by marking it as sealed. This will prevent any derived classes from being created, as the sealed class cannot be used as a parent class.
18. What is a base class in C#?
A base class is a class from which other classes can be derived. It is the parent class of a derived class, and the derived class will take on all the properties and methods of the base class.
19. What is the purpose of the new keyword in C#?
The new keyword is used to create a new instance of a class. It is typically used in combination with the constructor of a class to create a new instance of the class.
20. What is the difference between a class and a struct in C#?
A class is a reference type, while a struct is a value type. This means that a class is passed by reference and is stored on the heap, while a struct is passed by value and is stored on the stack. Additionally, classes have the ability to have methods and properties, while structs cannot.
Tips on Preparing for a Inheritance Interview
- Make sure you are familiar with the deceased’s will and estate plan.
- Gather and organize all relevant paperwork, including any bank statements, tax returns, deeds and contracts.
- Review the deceased’s estate plan for any special instructions and contingencies.
- Familiarize yourself with recent changes in estate law that could affect the inheritance.
- Bring a list of questions to the interview so that you can be prepared to ask questions and get clarification.
- If you are meeting with an attorney, be sure to bring a copy of any estate planning documents.
- Prepare to discuss how you plan to use your inheritance and how it will impact your financial goals.
- Be prepared to discuss any special requests the deceased may have made in their will.
- Be sure to take detailed notes during the interview so you can refer to them later.
- Ask if you can speak with the executor of the estate to ask additional questions.
- Ask for an explanation of any tax implications related to the inheritance.
- Ask if there is any special advice that the executor would like to share.
- Ask for an estimate of when you can expect to receive your inheritance.
- Be sure to bring any letters of instruction or notes from the deceased that you may have.
- Ask for a timeline of when the executor expects to wrap up the estate.
Conclusion
Inheritance is a powerful and important concept in object- oriented programming that allows developers to create and maintain applications more quickly and efficiently. With proper use of inheritance, an object can be created with all its characteristics without having to define each one separately. It can be used to simplify the process of maintaining and developing applications by helping to create classes that share common attributes and methods. This article has outlined some of the common interview questions that are related to inheritance and provided some sample answers to these questions. Understanding this concept is essential for any aspiring software developer and can help to make the development process more organized and productive.