Hashmaps are an important data structure used in programming. They provide quick and efficient ways of dealing with large amounts of data. They are a great resource for developers as they help speed up the process of finding and manipulating data. As such, they are often used in interviews to test a developer’s knowledge and skills. This article provides a comprehensive list of hashmap interview questions and answers.
The questions in this article cover all aspects of hashmaps, from the basics of hashmaps to the more complex topics. We will cover topics such as creating, searching, adding, and removing elements from a hashmap, as well as the different types of hashmaps, and how to implement them. We’ll also provide sample code snippets to help you answer the questions.
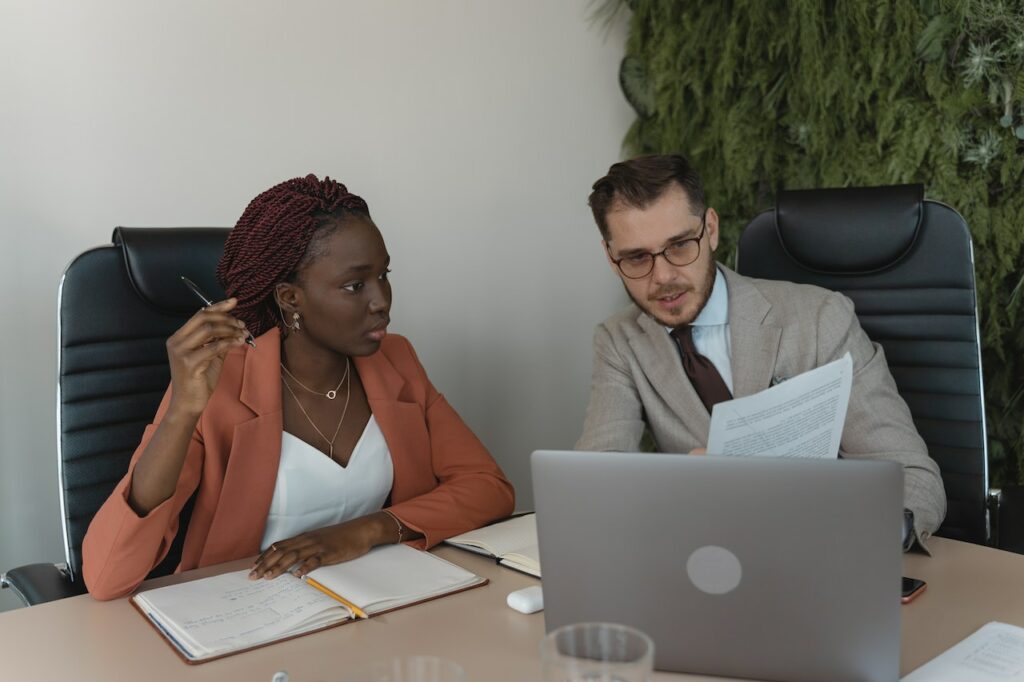
Hashmaps are an important part of any coding interview. Being able to demonstrate your knowledge and skills of hashmaps could be the difference between landing the job or not. That’s why it’s important to be prepared for any possible hashmap questions that may be asked. This article will provide everything you need to know to ace your hashmap interview.
Overview of Hashmap Interview Process
The HashMap interview process typically involves several steps depending on the employer. The typical process generally starts with an initial phone screen, which is used to determine if the applicant has the qualifications for the job. This could include questions about the applicant’s experience and background, as well as skills related to the specific HashMap position.
The next step in the interview process is usually an in- person interview. This interview will usually consist of two parts: a technical interview and a behavioral interview. The technical interview is a discussion of the applicant’s knowledge and experience with HashMap and related technologies, such as Java and JavaScript. During the behavioral interview, the hiring manager will ask questions to gain insight into the applicant’s abilities to work on a team, as well as their ability to take initiative and solve problems.
The final step in the interview process is an assessment. This assessment is typically an online test, or a coding challenge. This test is used to measure the applicant’s understanding of HashMap, as well as how well they can apply their knowledge to solve specific problems.
Depending on the employer, the interview process may also include additional steps such as a group interview or a second round of technical interviews. This additional step is used to further evaluate the applicant’s skills and knowledge, as well as their ability to work in a team environment. After these steps have been completed, the applicant will typically receive an offer or rejection letter.
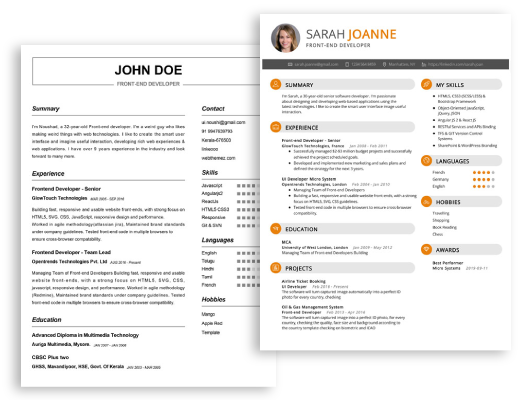
Start building your dream career today!
Create your professional resume in just 5 minutes with our easy-to-use resume builder!
Be sure to check out our resume examples, resume templates, resume formats, cover letter examples, job description, and career advice pages for more helpful tips and advice.
Top 20 Hashmap Interview Questions and Answers
1. What is a Hashmap?
A Hashmap is a type of data structure that is commonly used in programming languages. It is a collection of key-value pairs, where each key is associated with a single value. Hashmaps are efficient for lookups, and are used to store and retrieve data quickly. Hashmaps are also known as associative arrays since they associate a key to a value.
2. How does a Hashmap work?
A Hashmap looks up data by using a hash function to calculate the index of the item in the collection. This index is then used to access the item’s value. The hash function takes the key as an argument and returns an integer, which is then used as the index. The hashmap stores the item’s value at the index returned by the hash function. When trying to retrieve an item, the hashmap uses the same hash function to calculate the index of the item and access its associated value.
3. What are the advantages of a Hashmap?
A Hashmap is a very efficient data structure for lookups since it is able to quickly retrieve an item based on its key. This makes Hashmaps ideal for applications that require fast lookups. Hashmaps are also dynamic, meaning they can grow and shrink as needed without the need to reallocate memory. This makes them suitable for applications where the data set is constantly changing.
4. What are the disadvantages of a Hashmap?
The main disadvantage of a Hashmap is that it is not ordered. The order of the items in the collection is based on the hash function used to calculate the indices. This can lead to unexpected results when accessing or modifying data in a Hashmap. Hashmaps are also not suitable for applications that require sorting or searching data since the data is not stored in any particular order.
5. What is collision in a Hashmap?
Collision is the term used to describe when two different keys map to the same index in a Hashmap. This can occur when the hash function used to calculate the indices is not designed properly or when the data set is too large for the hash function to handle. When a collision occurs, the Hashmap cannot store both values in the same index and must choose which value to store.
6. How can collisions be avoided in a Hashmap?
Collisions can be avoided in a Hashmap by designing a hash function that is able to handle the data set and by increasing the size of the Hashmap. A good hash function is one that distributes the keys evenly throughout the Hashmap and minimizes the chances of collisions. Increasing the size of the Hashmap also helps since there are more indices to store the data in.
7. What is chaining in a Hashmap?
Chaining is a technique used to handle collisions in a Hashmap. If a collision occurs, the Hashmap stores the second value in a linked list at the same index as the first value. This allows both values to be stored without losing any data. Chaining also makes it possible to store multiple values in the same index, which can be useful in certain applications.
8. What is the difference between a Hashmap and a Hashtable?
The main difference between a Hashmap and a Hashtable is that the Hashmap allows null values to be stored, while the Hashtable does not. A Hashmap also uses a hash function to calculate the index of an item, while a Hashtable uses a table lookup. A Hashmap is also unsynchronized, while a Hashtable is synchronized, meaning that only one thread can access it at a time.
9. How do you iterate over a Hashmap?
There are several ways to iterate over a Hashmap. The most common way is to use the entrySet() method, which returns a Set of the Hashmap’s key-value mappings. The entrySet() method can then be used with a for-each loop to iterate over the Hashmap’s entries. Another way to iterate over a Hashmap is to use the keySet() method, which returns a Set of the Hashmap’s keys. These keys can then be used to access the associated values in the Hashmap.
10. How do you sort a Hashmap?
A Hashmap cannot be sorted directly since it does not store its data in any particular order. However, it is possible to sort a Hashmap by creating a new Map or List and populating it with the data from the Hashmap, sorted according to the desired criteria. The sorted Map or List can then be used instead of the Hashmap.
11. What is the Big O notation for a Hashmap?
The Big O notation for a Hashmap is O(1) for both insertion and lookup. This means that the time taken for insertion and lookup is constant and does not change with the size of the Hashmap.
12. How do you get the size of a Hashmap?
The size of a Hashmap can be retrieved using the size() method. This method returns an integer representing the number of items in the Hashmap.
13. How do you delete an element from a Hashmap?
An element can be deleted from a Hashmap using the remove() method. This method takes the key of the item to be removed as an argument and returns the value associated with that key.
14. How do you check if a key exists in a Hashmap?
A key can be checked for existence in a Hashmap using the containsKey() method. This method takes the key to be checked as an argument and returns a boolean value indicating whether the key exists in the Hashmap or not.
15. What is the best way to iterate over a Hashmap?
The best way to iterate over a Hashmap is to use the entrySet() method, which returns a Set of the Hashmap’s key-value mappings. The entrySet() method can then be used with a for-each loop to iterate over the Hashmap’s entries. This is the most efficient way to iterate over the Hashmap since it does not require the keys to be accessed separately.
16. How do you clear a Hashmap?
A Hashmap can be cleared using the clear() method. This method removes all the items from the Hashmap and sets the size to 0.
17. How do you copy a Hashmap?
A Hashmap can be copied using the putAll() method. This method takes another Hashmap as an argument and copies all of its key-value pairs into the target Hashmap. The target Hashmap must have enough space to store the items from the source Hashmap.
18. How do you convert a Hashmap to an array?
A Hashmap can be converted to an array using the toArray() method. This method takes an array as an argument and copies the items from the Hashmap into the array. The array must be large enough to store the items from the Hashmap.
19. How do you merge two Hashmaps?
Two Hashmaps can be merged using the putAll() method. This method takes another Hashmap as an argument and copies all of its key-value pairs into the target Hashmap. If the target Hashmap already contains an item with the same key, the new value will overwrite the existing one.
20. How do you check if a Hashmap is empty?
A Hashmap can be checked for emptiness using the isEmpty() method. This method returns a boolean value indicating whether the Hashmap is empty or not.
Tips on Preparing for a Hashmap Interview
- Become familiar with the syntax and common usages of Hashmap.
- Review the key differences between Hashmap and other data structures.
- Study the complexities of operations such as insertion, deletion, searching and traversal.
- Practice writing code snippets to insert, delete, search and traverse Hashmap elements.
- Understand how Hashmap handles collision and how to use hashing algorithms for better performance.
- Be aware of the various implementations of Hashmap and their differences.
- Prepare to answer questions about the methods and APIs of Hashmap.
- Understand what can happen when the size of Hashmap exceeds capacity.
- Understand the time and space complexities of different Hashmap operations.
- Be prepared to explain the differences between Hashmap and other data structures such as a tree.
- Practice answering questions about the advantages and disadvantages of using a Hashmap.
- Be prepared to explain how Hashmap can be used to improve performance of an application.
- Understand how to use the different Hashmap APIs and the proper way to use each one.
- Practice writing code snippets to include Hashmap in existing code.
- Understand how to optimize Hashmap operations for better performance.
Conclusion
Hashmaps are an essential tool for any programmer, and mastering the skill is key for both experienced and beginner programmers. Having a good understanding of hashmaps and being able to answer related interview questions is essential for any programmer looking to get a job. The above blog post provides a comprehensive list of hashmap related interview questions, as well as detailed answers that can help any programmer prepare for a job interview. With the right preparation and practice, any programmer can get a job they want while demonstrating their knowledge of hashmaps.