Constructor in Java is an important topic of discussion for any Java interview. As a Java developer, you should have a strong command over the topics like defining a constructor, understanding the types of constructors, the role of Constructors in inheritance, and writing a constructor in Java.
In this blog, we will discuss the most frequently asked Constructor in Java Interview Questions & Answers. As a Java developer, it is very important to understand the functionality and use of the constructor. Knowing the answers to commonly asked Java Interview questions related to Constructors will give you an edge over other candidates.
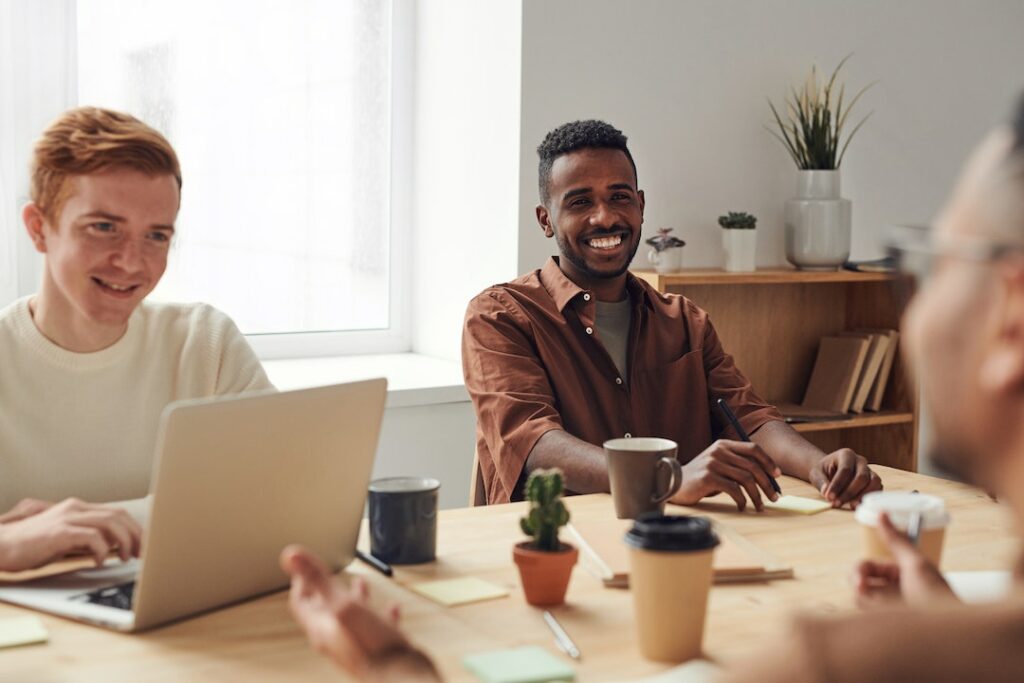
All the answers provided to the Constructor in Java Interview Questions & Answers are thoroughly researched and provide an accurate insight into the questions. We will try to cover all the important concepts related to Java constructors, including defining a constructor, understanding the types of constructors, the role of Constructors in inheritance, and writing a constructor in Java. Furthermore, we will also discuss a few frequently asked Java constructor interview questions and answers, which will give you an edge over other candidates.
This blog is a comprehensive guide, which will help you to understand the concept of Constructor in Java and the frequently asked interview questions related to it. Through the answers to Java constructor interview questions given in this blog, you will be able to confidently answer any Java interview questions that you may come across.
Overview of Constructor in Java Interview Process
The constructor in Java interview process is an important component of the hiring of a Java programmer. A constructor is a special type of method in Java that is used to create an object, either by instantiating a new instance of the object or by initializing an existing instance. It is typically used to set an initial state of the object or to perform some initialization tasks. Constructors are generally used to create a new instance of a class, and are often used to set the values of member variables.
The interviewer will be looking for a candidate who is familiar with the different types of constructors and can explain their use. The candidate should also be able to demonstrate their understanding of the syntax of constructors, as well as their ability to write code that correctly uses constructors in a variety of scenarios.
The interviewer will likely ask the candidate to explain the different types of constructors, such as the default constructor, parameterized constructor, and the copy constructor. They may also be asked to describe the differences between constructors and other methods, such as setters and getters. The interviewer may also ask the candidate to provide code examples of how they would use constructors in different scenarios.
The candidate should be familiar with the syntax of constructors, as well as any related concepts, such as overloading and how to properly use the constructor to initialize a class. They should also be prepared to discuss any potential issues that may arise when using constructors, such as memory leaks or potential performance issues.
In sum, the constructor in Java interview process is an important part of the hiring process. The interviewer will be looking for a candidate who is knowledgeable about the types of constructors, their syntax, and how to use them in a variety of scenarios. The candidate should be prepared to discuss any potential issues that may arise when using constructors.
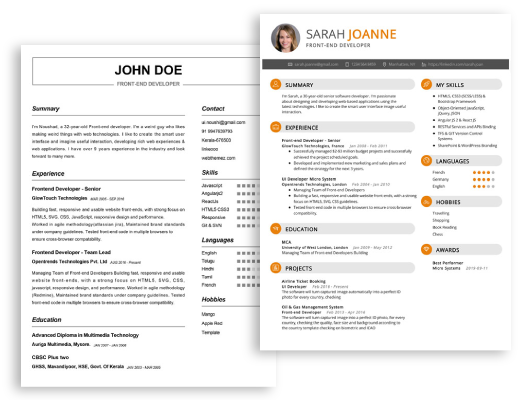
Start building your dream career today!
Create your professional resume in just 5 minutes with our easy-to-use resume builder!
Be sure to check out our resume examples, resume templates, resume formats, cover letter examples, job description, and career advice pages for more helpful tips and advice.
Top 18 Constructor in Java Interview Questions and Answers
Q1. What is a Constructor in Java?
A constructor in Java is a special type of method that is used to initialize the state of an object. It is called when an object of a class is created and has the same name as the class. Constructors don’t return any value, not even void, and they can not be inherited. They are primarily used to set initial values for the instance variables of an object and to perform any other startup procedures required to create a fully formed object.
Q2. What are the different types of Constructors in Java?
There are three types of constructors in Java: default, no-arg, and parameterized. The default constructor is used when no arguments are passed to the constructor, and it sets the instance variables of the object to their default values. The no-arg constructor is used when no arguments are passed to the constructor, and it sets the instance variables of the object to the values specified in the constructor. The parameterized constructor is used when arguments are passed to the constructor, and it sets the instance variables of the object to the values specified in the constructor.
Q3. What is the purpose of a Constructor in Java?
The primary purpose of a constructor in Java is to initialize the state of an object. It is called when an object of a class is created and allows the programmer to set initial values for the instance variables of the object. Constructors also allow the programmer to perform any other startup procedures required to create a fully formed object.
Q4. Does a Constructor have a return type?
No, a Constructor does not have a return type. Constructors must not have a return type, not even void, and they can not be inherited.
Q5. Can a Constructor be overloaded in Java?
Yes, a constructor can be overloaded in Java. When a constructor is overloaded, multiple versions of the constructor are created with different parameters. This allows a programmer to create objects in different ways, depending on the parameters passed to the constructor.
Q6. What is the difference between a Constructor and a Method?
The main difference between a constructor and a method is that a constructor is used to initialize the state of an object, while a method is used to perform an action or task. Additionally, a constructor does not have a return type, while a method must have a return type.
Q7. What is the difference between a Constructor and an Initializer Block?
The main difference between a constructor and an initializer block is that a constructor is used to initialize the state of an object, while an initializer block is used to initialize static variables of a class. Additionally, an initializer block is executed when the class is loaded, while a constructor is executed when an object is created.
Q8. What is the use of the super keyword in a Constructor?
The super keyword is used in a constructor to call the constructor of the superclass. This allows the subclass constructor to inherit the instance variables and methods of the superclass.
Q9. What is a Copy Constructor in Java?
A copy constructor in Java is a constructor that takes an object of the same class as a parameter and creates a copy of the object. This allows the programmer to create a copy of an object without having to manually set each field of the object.
Q10. What is the difference between a Constructor and a Copy Constructor?
The main difference between a constructor and a copy constructor is that a constructor is used to initialize the state of an object, while a copy constructor is used to create a copy of an existing object. Additionally, a copy constructor takes an object of the same class as a parameter, while a constructor does not take any parameters.
Q11. What is the purpose of the constructor in Java?
The main purpose of the constructor in Java is to provide a way to initialize objects when they are created. Constructors can also be used to set values for instance variables, such as size, type, color, etc., during object creation.
Q12. What is the syntax of a constructor in Java?
The syntax of a constructor in Java is as follows:public ClassName (parameter list) { // body of constructor }
The constructor must have the same name as the class, and it must not have any return type.
Q13. What are the types of constructors in Java?
There are two types of constructors in Java:
- Default constructor: A default constructor is a constructor without any parameters. It is used to create objects with the default values for instance variables.
- Parameterized constructor: A parameterized constructor is a constructor with parameters. It is used to create objects with specific values for instance variables.
Q14. How do you call a constructor in Java?
A constructor in Java can be called using the new keyword. For example, to create an object of a class named “MyClass” you would use the following syntax: MyClass myObject = new MyClass();
Q15. What is the difference between a constructor and a method in Java?
The main difference between a constructor and a method in Java is that a constructor is used to create an instance of a class, while a method is used to perform an action. A constructor does not have a return type, while a method does.
Q16. How do you call a superclass constructor in Java?
A superclass constructor can be called using the super keyword. For example, to call the superclass constructor of a class named “MyClass” you would use the following syntax: super();
Q17. Can a constructor be private in Java?
Yes, a constructor in Java can be private. A private constructor is used to prevent other classes from creating an instance of the class.
Q18. What is the purpose of the this keyword in Java?
The this keyword in Java is used to refer to the current instance of a class. It is used to access instance variables and methods of the current object. It can also be used to call another constructor of the same class.
Tips on Preparing for a Constructor in Java Interview
- Understand the fundamentals of Java, including the basic language features, syntax, and common libraries.
- Familiarize yourself with the Java Constructor concept, including what it is and how to use it.
- Practice coding challenges that use the Java Constructor concept.
- Learn and practice object- oriented programming principles and design patterns.
- Understand the different types of constructors and their uses.
- Prepare to explain your code, as the interviewer may ask questions about it.
- Research the company and their product, if possible, to show your knowledge and interest.
- Get plenty of rest the night before and arrive to the interview early.
- Dress professionally and be sure to bring extra copies of your resume and portfolio.
- Show enthusiasm about the job opportunity and confidence in your skills.
- Don’t be afraid to ask questions and seek clarification if needed.
- Demonstrate the ability to think logically and solve problems.
- Be able to explain why you chose a particular solution over another.
- Be able to explain and provide examples of the Constructor concept.
- Stay up- to- date with current trends and technologies in the Java world.
Conclusion
In conclusion, the constructor in Java is a very important concept that every Java developer should be familiar with. Constructors are used to create objects with the desired properties and methods, and to assign values to the variables of the class. When preparing for a Java interview, it is important to understand how to create a constructor, how to call it, and how to use it to initialize the fields of an object. Being prepared and knowledgeable on the subject of constructors will ensure that you are well- equipped to answer any questions related to the topic during a Java interview.