Inheritance is an important concept in Java programming. It is a way of defining relationships between a superclass and its subclasses. Through inheritance, subclasses can inherit the properties and methods of their superclasses, making it easier to reuse code. This makes inheritance an important concept to understand for any Java programmer.
Interviewers may ask questions about inheritance to test a candidate’s knowledge of the language. These questions may range from basic questions about inheritance syntax to more complex questions about the practical implications of using inheritance in code. It is important for any candidate to be prepared for these types of questions.
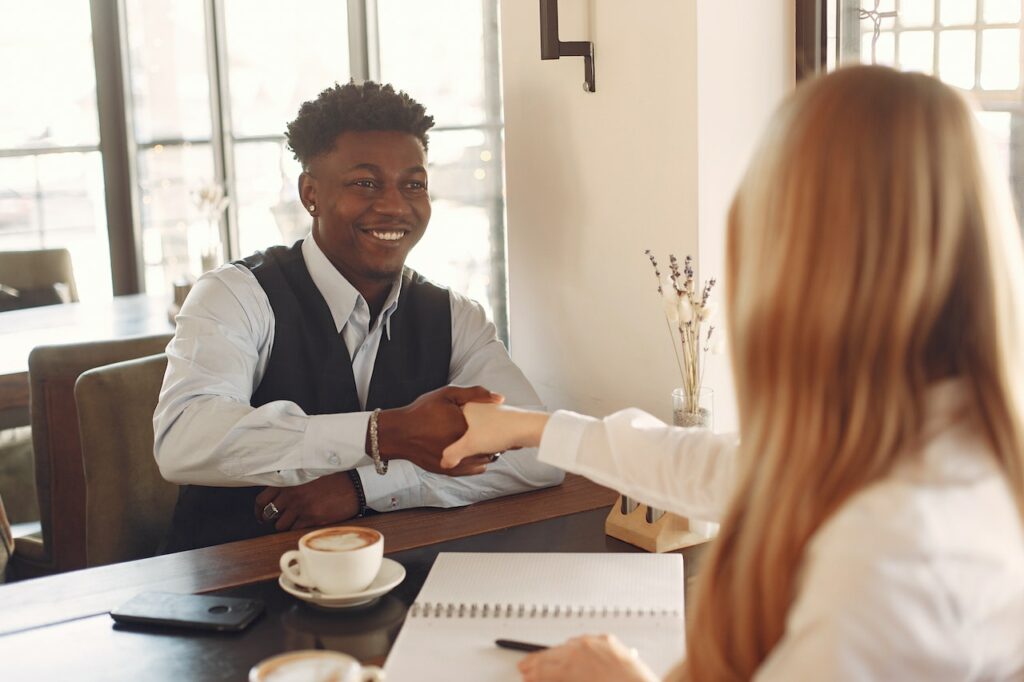
In this blog, we will explore some common Java inheritance interview questions and answers. We will look at questions related to inheritance syntax, the advantages of inheritance, and best practices when using inheritance in Java. We will also provide examples of code to illustrate inheritance concepts. With this information, you will be prepared to answer inheritance-related questions confidently in your next interview.
Overview of Java inheritance Interview Process
Java inheritance is an important part of the Java language and is often a topic covered in interviews. Interviewers may ask about Java inheritance concepts, such as what it is and how it works. They may also ask specific questions about how inheritance works in a Java program, such as how to override a method or how to use the super keyword. Additionally, interviewers may ask more complex questions about inheritance, such as how to use multiple inheritance and how to choose between inheritance and composition.
In a Java inheritance interview, the interviewer will likely start by asking general questions about inheritance like what it is and how it works. The candidate should be able to explain the different types of inheritance and how they are implemented. They should also show an understanding of how to override methods and how to use the super keyword.
The interviewer may then ask specific questions about how inheritance is used in a Java program. This could include questions about how to create a subclass, how to use the super keyword, and how to override methods. The candidate should be able to provide examples and explain the implications of each type of inheritance.
Finally, the interviewer may ask more complex questions about inheritance, such as how to use multiple inheritance and how to choose between inheritance and composition. The candidate should be able to demonstrate an understanding of how these concepts work and how they can be applied to a given project. They should also be familiar with the trade- offs between each type of inheritance and when they are best used.
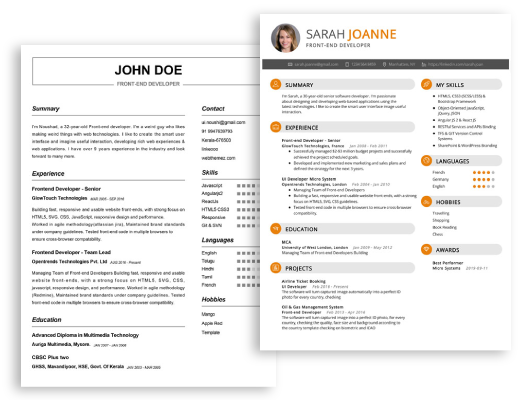
Start building your dream career today!
Create your professional resume in just 5 minutes with our easy-to-use resume builder!
Be sure to check out our resume examples, resume templates, resume formats, cover letter examples, job description, and career advice pages for more helpful tips and advice.
Top 18 Java inheritance Interview Questions and Answers
1. What is Inheritance in Java?
Inheritance in Java is the process by which one object or class acquires the properties of another object or class. It is an important feature of object-oriented programming that allows the creation of a class hierarchy, which can be used to create new classes that share the characteristics of existing classes while adding their own unique features. Through inheritance, a class can be derived from another class, which is known as the parent or base class. The derived class, known as the child or subclass, inherits the fields and methods of the parent class.
2. What is the purpose of inheritance in Java?
Inheritance in Java allows us to reuse existing code. It makes it easier to maintain and upgrade existing code, as any changes made to the parent class will be automatically applied to the child classes. It also helps us to add new functionality by creating subclasses that can extend the functionality of the parent class. Finally, inheritance improves code readability and organization by making the code easier to understand and navigate.
3. What is the difference between inheritance and composition in Java?
The main difference between inheritance and composition in Java is that inheritance refers to the relationship between classes, while composition refers to the relationship between objects. Inheritance is a mechanism by which one class can extend the functionality of another class, while composition is a mechanism by which one object can contain or use another object.
4. What are the different types of inheritance in Java?
There are four different types of inheritance in Java.
The first is single inheritance, which is when a class extends a single parent or base class.
The second is multiple inheritance, which is when a class extends more than one parent or base class.
The third is hierarchical inheritance, which is when multiple classes are extended from a single parent or base class.
The fourth is multilevel inheritance, which is when a class extends a class that is derived from another class.
5. What is the super keyword in Java?
The super keyword in Java is used to refer to the immediate parent class or superclass. It can be used to access the parent class’ constructors, methods, and variables. The super keyword is also used to invoke the parent class’ version of an overridden method.
6. What is method overriding in Java?
Method overriding in Java is a feature that allows a subclass or child class to provide a specific implementation of a method that is already defined by one of its superclasses or parent classes. It is a way of making the code more flexible by allowing the same method to have different implementations in different classes.
7. What is a constructor in Java?
A constructor in Java is a special type of method that is used to initialize an object when it is created. Constructors have the same name as the class and are called when an object of the class is created. Constructors can have parameters, which can be used to provide initial values for fields in the class.
8. What is the difference between overloading and overriding in Java?
The difference between overloading and overriding in Java is that overloading allows a method to have multiple implementations with different parameters, while overriding allows a subclass to provide a specific implementation of a method that exists in a parent or superclass.
9. What is polymorphism in Java?
Polymorphism in Java is the ability of an object to take on multiple forms. It is a feature of object-oriented programming that allows a single object to have different implementations of a method depending on the context in which it is called.
10. What is an abstract class in Java?
An abstract class in Java is a special type of class that cannot be instantiated. An abstract class is used to provide a common interface or set of features to be shared by multiple classes. An abstract class can have both abstract and concrete methods, which can be overridden by subclasses.
11. How is multiple inheritance implemented in Java?
Multiple inheritance in Java is implemented using interface. A class can implement multiple interfaces, and each interface can have its own set of methods and variables. The methods and variables defined in an interface are inherited by any class that implements it.
12. What is a final class in Java?
A final class in Java is a special type of class that cannot be extended or subclassed. A final class can still be instantiated, and its methods and variables can still be used. Final classes are used to provide a level of security, as they cannot be overridden or extended by other classes.
13. What is the difference between a final class and an abstract class in Java?
The difference between a final class and an abstract class in Java is that a final class cannot be extended or subclassed, while an abstract class can be extended by other classes. A final class can still be instantiated, while an abstract class cannot.
14. What is an abstract method in Java?
An abstract method in Java is a method that is declared in an abstract class but does not have an implementation. Abstract methods must be overridden by any class that extends the abstract class.
15. What is an interface in Java?
An interface in Java is a collection of abstract methods and constants that define a set of common behaviors for all classes that implement the interface. An interface is like a contract between the implementer and the user of the interface.
16. What is the difference between an interface and an abstract class in Java?
The main difference between an interface and an abstract class in Java is that an interface can only contain abstract methods and constants, while an abstract class can contain both abstract and concrete methods. An abstract class can also contain instance variables, while an interface cannot.
17. What is the purpose of the Object class in Java?
The Object class in Java is the parent class of all other classes. It is the root of the class hierarchy and is used to provide some common methods and fields to all classes, such as hashCode() and toString(). The Object class is also used to define the equals() and clone() methods.
18. What is the difference between a class and an object in Java?
The difference between a class and an object in Java is that a class is a blueprint or template from which objects are created, while an object is an instance of a class. A class can have multiple objects, while an object can only be associated with one class.
Tips on Preparing for a Java inheritance Interview
- Take the time to review the fundamentals of Java inheritance, such as the structure of classes, how to create and extend a class, and how to use polymorphism.
- Understand the different types of inheritance and how they interact with each other.
- Have a basic understanding of UML (Unified Modeling Language) and how to create class diagrams.
- Practice coding examples of different inheritance scenarios.
- Be prepared to explain how multiple inheritance works and the potential complexities it can bring.
- Be able to explain how constructors work and how they are affected by inheritance.
- Understand the concept of overriding and how to use it with inheritance.
- Understand how the visibility of class members is affected by inheritance.
- Be able to explain the purpose of an abstract class and why it is used.
- Demonstrate your knowledge of the differences between superclass and subclass.
- Understand the basics of using the keyword ‘super’ in Java.
- Have an understanding of the Java Reflection API and how it can be used to inspect the class hierarchy of a program.
- Be prepared to answer questions about the use and purpose of the ‘final’ keyword.
- Understand the concept of polymorphism and how it is used in Java.
- Have a thorough understanding of how the Java compiler handles inheritance.
Conclusion
Learning the fundamentals of Java inheritance is a key part of becoming a successful Java programmer. By familiarizing yourself with these common Java inheritance interview questions and answers, you can be better prepared to answer questions during a job interview. Knowing the basics of Java inheritance can help you understand the concepts of object- oriented programming and can help you write better programs. With a little practice and some guidance, you can become an expert on Java inheritance and be able to answer any related interview questions with confidence.