Oops C# Interview Questions & Answers is an essential guide for all software developers aspiring to crack C# coding interviews. As C# is one of the most popular programming languages, the demand for C# developers is consistently high in the industry.
Having a solid knowledge of basic Oops concepts and its implementation in C# is essential to crack C# coding interviews. As C# is an object-oriented language, a sound understanding of Oops concepts such as inheritance, abstraction, encapsulation, polymorphism and their real-world implementation is important to be successful in the C# coding interviews.
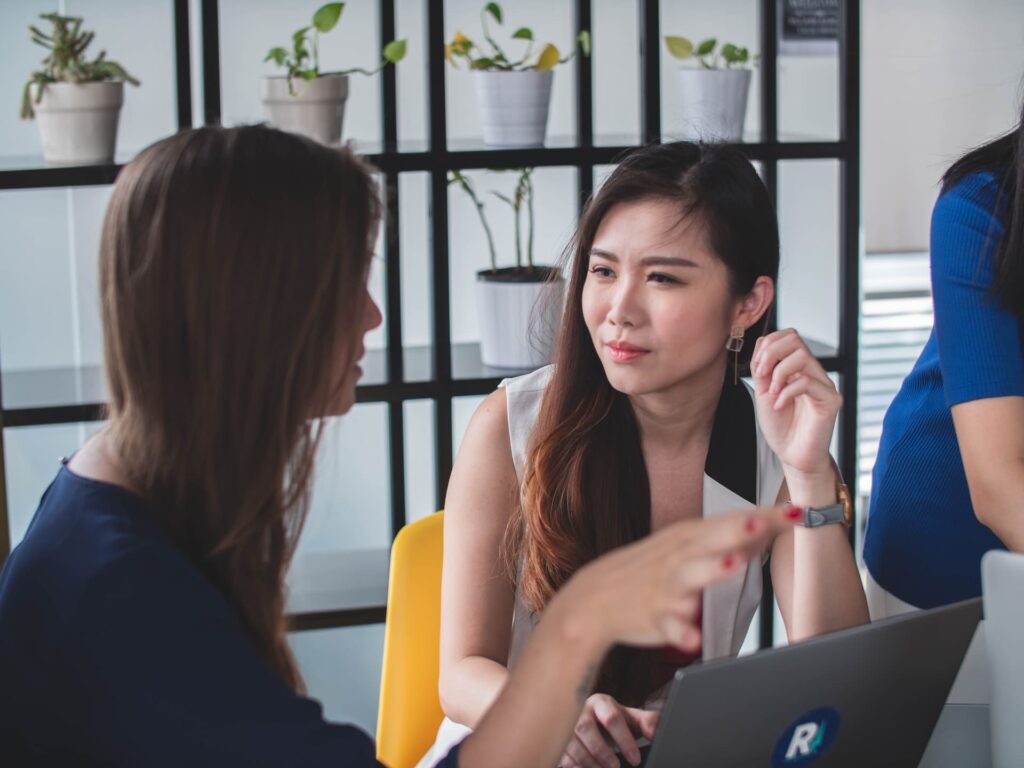
This blog post is designed for all those software developers who are looking for the best Oops C# Interview Questions & Answers. Here, we have compiled a comprehensive list of the most commonly asked questions in C# coding interviews. We have also provided detailed answers to each question which will help you to understand the concept better and develop a deeper understanding of the Oops concepts in C#.
We have also provided tips and strategies to ensure that you are well-prepared to tackle the C# coding interviews. These tips and strategies will help you to gain a competitive edge in the C# coding interviews. We recommend that you go through the questions and answers multiple times to get a good understanding of the concepts. This will help you to ace the C# coding interviews and stand out from the crowd.
Overview of Oops C# Interview Process
The interview process for a position in which the candidate is expected to know object- oriented programming with C# can be daunting, but it doesn’t have to be. As with any job interview, it’s important to go in prepared and understand what areas you may be asked about.
The interviewer will likely start by asking about your background and experience as it pertains to OOP and C#. They’ll be looking to get a sense of your understanding of the fundamentals and if you can think on your feet. They may ask questions such as, “What is the difference between an interface and an abstract class?” or “Can you explain the concept of inheritance?”.
Next, the interviewer may look to assess your problem- solving skills. To do this, they may give you a code problem and ask you to solve it. They’ll want to see how you approach the problem and break it down into manageable steps. They may also ask you to explain how a particular piece of code works.
It’s likely that the interviewer will also ask you to demonstrate your knowledge of the .NET framework, as well as core libraries such as LINQ and Entity Framework. Be prepared to explain how each library works and provide a few examples of how you’ve used them.
The interview process should wrap up with a discussion of your experience in the workplace. This may include questions about how you interact with teams, handle difficult tasks, and adhere to company policies. The interviewer may also ask you questions to gauge if you are the right fit for their company culture.
Overall, the interview process for a C# OOP position can be quite rigorous. It is important to be well- prepared and practice answering potential questions ahead of time. Doing so will ensure you present yourself in the best possible way while communicating your knowledge and experience effectively.
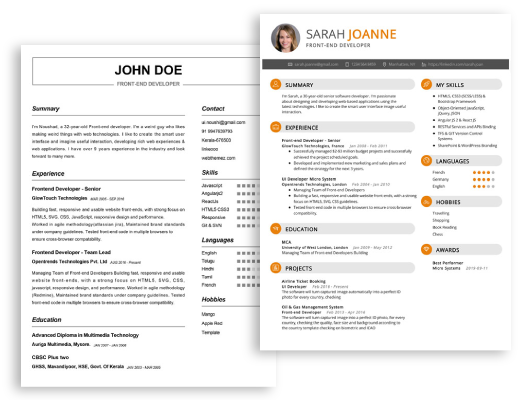
Start building your dream career today!
Create your professional resume in just 5 minutes with our easy-to-use resume builder!
Be sure to check out our resume examples, resume templates, resume formats, cover letter examples, job description, and career advice pages for more helpful tips and advice.
Top 20 Oops C# Interview Questions and Answers
1. What is OOPS ?
Object-Oriented Programming (OOP) is a programming paradigm based on the concept of objects, which can contain data, in the form of fields, and code, in the form of procedures, often known as methods. OOP languages are designed to overcome the shortcomings of procedural languages. It allows the developer to create objects that can be reused in different parts of a program, which helps in reducing the complexity of the code.
2. What is a class in OOPS ?
A class is a blueprint for creating objects (a particular data structure), providing initial values for state (member variables or attributes), and implementations of behavior (member functions or methods). It allows for the definition of characteristics and behavior of the object. Classes can also define relationships between different objects and can be composed of other classes.
3. What are the different access specifiers in C# ?
The different access specifiers in C# are public, private, protected, internal, and protected internal.
4. What is inheritance in C# ?
Inheritance allows a class to use the properties and methods of another class. Inheritance allows for code reuse and a hierarchical structure to be formed within a program. A derived class, or child class, can access all the members of a parent class, or base class, except those marked as private.
5. What is polymorphism in C# ?
Polymorphism allows objects of different types to be treated the same way. It allows for the same code to be used with different types of objects through dynamic binding. This means that an object can take on different forms depending on the context in which it is used, and can be treated as either an object of its parent class or an object of its derived class.
6. What is an abstract class in C# ?
An abstract class is a class that is declared with the ‘abstract’ keyword. It is a base class that cannot be instantiated and is used as a template for other classes. A class may be declared abstract even if it does not contain any abstract methods, in which case it cannot be instantiated.
7. What is an interface in C# ?
An interface is a set of related methods and properties that can be implemented by a class. Interfaces are declared with the ‘interface’ keyword and contain only the signatures of the methods and properties, not the implementation.
8. What is an abstract method in C# ?
An abstract method is a method that is declared with the ‘abstract’ keyword and does not have an implementation. Abstract methods must be implemented by any class that inherits from the abstract class that contains the abstract method.
9. What is a delegate in C# ?
A delegate is a type that refers to a method or a function. Delegates are used to represent a method and can be used to invoke that method at runtime. Delegates are similar to function pointers in C and C++.
10. What is an event in C# ?
An event is a message sent by an object to signal the occurrence of an action. Events are declared with the ‘event’ keyword and can be used to invoke methods in the class that declares the event. Events are often used to notify other classes that something has occurred.
11. What is the difference between a class and an object in C# ?
A class is a template or blueprint from which objects are created. A class defines the properties and behavior of objects of that class. An object is an instance of a class and is created at runtime. An object contains its own data and can be manipulated independently of other objects of the same class.
12. What is a namespace in C# ?
A namespace is a logical grouping of classes and other types. Namespaces are used to organize code and prevent name collisions between different classes.
13. What is a constructor in C# ?
A constructor is a special method that is called when an object is created. It initializes the data members of the object and can be used to set the state of the object.
14. What is a destructor in C# ?
A destructor is a special method that is called when an object is destroyed. It releases any resources that were allocated by the object and can be used to clean up resources before the object is destroyed.
15. What is an indexer in C# ?
An indexer is a special type of property that allows an array-like syntax to be used to access elements of a class. Indexers are similar to array elements and allow the class to be indexed like an array.
16. What is an extension method in C# ?
An extension method is a special type of static method that can be used to extend the functionality of a class without having to modify the class. Extension methods are declared with the ‘this’ keyword and can be called on an instance of the class that they are extending.
17. What is an anonymous type in C# ?
An anonymous type is a type that does not have a name and can only be used in the scope of a single method. Anonymous types are typically used for creating objects that contain a set of properties that are not known until runtime.
18. What is a lambda expression in C# ?
A lambda expression is an anonymous function that can be used to create a delegate or expression tree. Lambda expressions can be used to reduce the amount of code that is needed to define a function, and can be used to simplify the syntax of LINQ queries.
19. What is a partial class in C# ?
A partial class is a class that is split into multiple files. Partial classes allow different parts of a class to be defined in different files and can be used to organize large classes into multiple files.
20. What is an anonymous method in C# ?
An anonymous method is an anonymous function that can be used to create a delegate. Anonymous methods allow a method to be defined without having to specify the method name or return type. Anonymous methods are typically used for defining a method that is only used once in a program.
Tips on Preparing for a Oops C# Interview
- Research the company thoroughly and understand their product, services and technology stack.
- Review the fundamentals of the C# language, including classes, objects, exception handling, generics, delegates and lambda expressions.
- Brush up on your knowledge of concepts such as inheritance, polymorphism, and abstraction.
- Understand the basics of the .NET framework, such as the Common Language Runtime (CLR), the .NET Base Class Library (BCL) and the CLR security model.
- Understand how to use the Visual Studio IDE for developing and debugging C# programs.
- Review the fundamentals of Windows Application Development, including user interface design and development, and database integration.
- Understand commonly used C# development techniques such as refactoring, unit testing and continuous integration.
- Practice coding questions in C# and understand the best practices for solving them.
- Review the basics of object- oriented design patterns and their implementation in C#.
- Be aware of the latest trends in C# development, such as the use of the asynchronous programming model and the Reactive Extensions (Rx).
- Be ready to discuss the pros and cons of various design decisions and the trade- offs between different approaches.
- Understand the concept of SOLID design principles and the principles of good software design and architecture.
- Understand the importance of version control systems, such as Git and Subversion, and the basics of continuous integration systems.
- Practice creating algorithms and data structures in C#.
- Have examples of projects that you have created using C# ready to discuss.
Conclusion
Overall, the C# language is one of the most popular and versatile programming languages in the world, and is a great choice for anyone looking to get started in computer programming. By becoming knowledgeable in C#, you can become a valuable asset to any organization. The C# interview questions and answers in this article can help you prepare for your next job interview and show employers that you are an experienced and knowledgeable programmer. Good luck!