Java Polymorphism is an important concept in object-oriented programming. It is the process of using a single interface to perform different tasks. This allows for the reuse of code and allows for more efficient programming.
Polymorphism is a feature of Java that allows one object to take on multiple forms. It is the ability of an object to take on different forms depending on the context. By polymorphism, it is possible to perform a single action in different ways. This allows you to write code that is cleaner and easier to read.
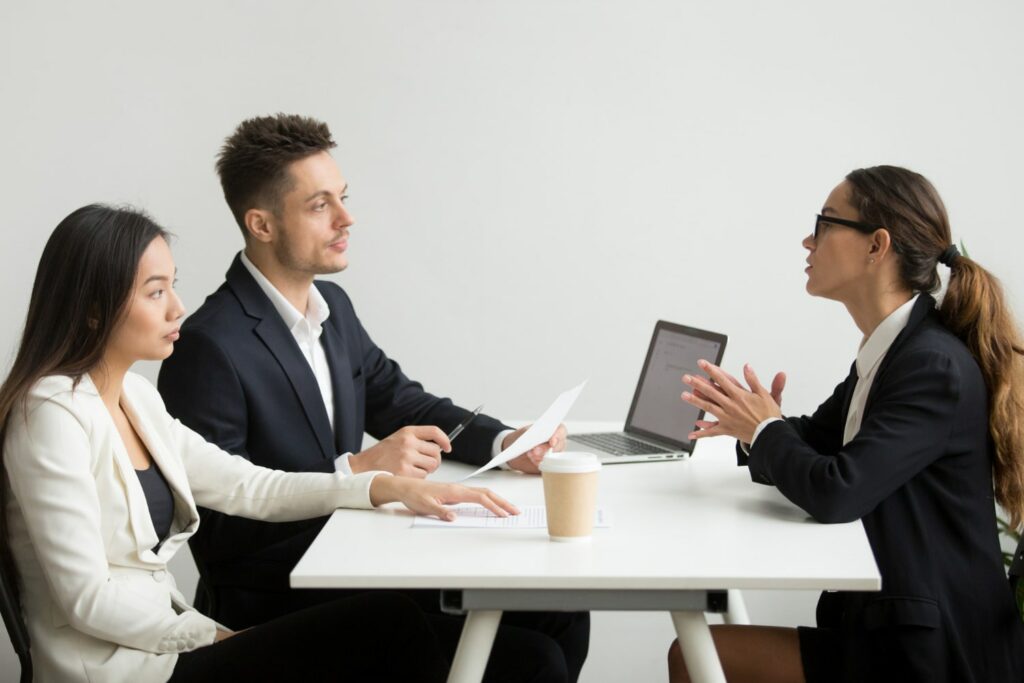
Java polymorphism is a concept that is used to re-use existing code, reduce complexity and improve code readability. By using this concept, we can create a single interface to perform multiple tasks. This makes it easier to understand and maintain applications. It is important to understand the concept of polymorphism in order to develop robust Java applications.
If you are preparing for a Java interview, it is important to be aware of the concept of polymorphism. In this article, we will discuss some of the common Java polymorphism interview questions and answers.
The questions will cover topics such as the advantages of polymorphism, how to implement polymorphism in Java, and how to use the polymorphic methods. We will also discuss the difference between static and dynamic polymorphism and the different types of polymorphism in Java.
By the end of this article, you will have a better understanding of the concept of polymorphism and be able to answer Java Interview questions about polymorphism confidently.
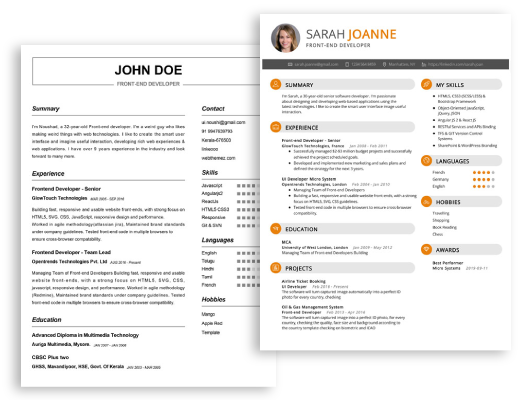
Start building your dream career today!
Create your professional resume in just 5 minutes with our easy-to-use resume builder!
Be sure to check out our resume examples, resume templates, resume formats, cover letter examples, job description, and career advice pages for more helpful tips and advice.
Top 15 Java Polymorphism Interview Questions and Answers
1. What is polymorphism in Java?
Polymorphism in Java is the ability of an object to take on multiple forms. It is an essential concept in object-oriented programming, where the same interface can be used to handle different underlying data objects. It allows for the same code to be used for different objects by substituting different implementations for the same interface. This enables a great deal of flexibility and code reuse.
2. What are the different types of polymorphism?
There are two types of polymorphism in Java: compile time polymorphism (static binding) and runtime polymorphism (dynamic binding). Compile time polymorphism is achieved through method overloading, while runtime polymorphism is achieved through method overriding.
3. What is method overloading in Java?
Method overloading is a type of polymorphism in Java where multiple methods in a class have the same name, but different parameters. This allows for the same code to be used for different objects by substituting different parameters for the same method name.
4. What is method overriding in Java?
Method overriding is a type of polymorphism in Java where a parent class method is overridden by a child class method. This allows for the same code to be used for different objects by substituting different implementations for the same interface.
5. What is the difference between method overloading and method overriding?
The difference between method overloading and method overriding is that method overloading is a type of polymorphism in Java where multiple methods in a class have the same name, but different parameters, while method overriding is a type of polymorphism in Java where a parent class method is overridden by a child class method.
6. How can polymorphism be achieved in Java?
Polymorphism in Java can be achieved through method overloading and method overriding. Method overloading is a type of polymorphism in Java where multiple methods in a class have the same name, but different parameters. Method overriding is a type of polymorphism in Java where a parent class method is overridden by a child class method.
7. Does Java support multiple inheritance?
No, Java does not support multiple inheritance. Java supports single inheritance, where a subclass inherits from a single superclass. This is due to the diamond problem, which can cause ambiguity when multiple inheritance is used.
8. What is the diamond problem in java?
The diamond problem in Java occurs when a subclass inherits from multiple superclasses, and there is ambiguity about which method or property is being called. This is due to the fact that the same method or property may be present in multiple superclasses, and the subclass will not know which one to use.
9. How is the diamond problem solved in Java?
The diamond problem is solved in Java by using single inheritance, where a subclass inherits from a single superclass. This ensures that there is no ambiguity about which method or property is being called, since there is only one superclass.
10. What is the purpose of the super keyword in Java?
The super keyword in Java is used to access the members of the superclass from the subclass. It is used to reference the parent class, and is often used in the context of method overriding.
11. What is the purpose of the final keyword in Java?
The final keyword in Java is used to indicate that a method, class, or variable cannot be overridden or changed. It is used to prevent subclasses from overriding methods, or to indicate that certain methods should not be changed.
12. What is the purpose of the abstract keyword in Java?
The abstract keyword in Java is used to indicate that a class or method is abstract. An abstract class is one that cannot be instantiated, and is used as a base class for other classes. An abstract method is a method that has no implementation, and must be overridden by the subclass.
13. What are the advantages of polymorphism in Java?
The advantages of polymorphism in Java include code reuse, flexibility, and extensibility. By being able to use the same code for different objects, code reuse is made much easier. Additionally, polymorphism allows for flexibility, as different implementations can be used for the same interface. Finally, polymorphism allows for extensibility, as new methods and classes can easily be added.
14. What is the difference between an interface and an abstract class in Java?
The main difference between an interface and an abstract class in Java is that an interface is a type of contract that defines a set of methods that must be implemented, while an abstract class is a class that cannot be instantiated and is used as a base class for other classes. Additionally, an interface is used to achieve polymorphism through method overriding, while an abstract class is used to achieve polymorphism through method overloading.
15. What is the relationship between polymorphism and inheritance in Java?
The relationship between polymorphism and inheritance in Java is that polymorphism is achieved through inheritance. This is because polymorphism allows for the same code to be used for different objects by substituting different implementations for the same interface, which is done through inheritance. For example, polymorphism can be achieved through method overloading, which is done by having multiple methods in a class with the same name, but different parameters. This is achieved by subclassing a class and overriding the parent class’s method.
Overview of Java Polymorphism Interview Process
Java polymorphism is a powerful feature of the Java programming language that enables objects of different types to share the same interface and behavior. It is an important concept in object- oriented programming and plays an integral role in achieving code reusability and extensibility. Polymorphism is implemented in Java using inheritance and overriding, where a parent class defines an interface or behavior and subclasses are able to extend or modify the behavior of the parent class.
Polymorphism refers to the ability of an object to take on different forms depending on the context in which it is used. In Java, polymorphism is implemented using inheritance and overriding. To achieve polymorphism, a class must define an interface or behavior and then subclasses can extend or modify the interface or behavior of the parent class. As a result, the same behavior can be applied to different objects of different types.
Polymorphism is a critical concept in Java programming and enables developers to write code that is both reusable and extensible. For example, a single method can be written to work with any type of object as long as the parameters of the method are defined correctly. This makes code maintenance easier as the same code can be used for different objects without the need for rewriting. It also makes debugging simpler as there are fewer lines of code to debug.
The interview process for a Java polymorphism position may involve a variety of tests, such as coding challenges, written exams, algorithm design tasks, and even whiteboard coding. The interviewer will usually begin by asking questions to determine the candidate’s understanding of the concepts of inheritance and overriding as they relate to polymorphism. The interviewer may then proceed to ask more detailed questions on the features and behavior of Java classes and objects, as well as how polymorphism can be used to achieve code reusability and extensibility.
Tips on Preparing for a Java Polymorphism Interview
- Be sure to understand the basics of polymorphism, including the concept of inheritance and method overriding.
- Understand the implications of implementing polymorphism in Java, such as how to handle different types of objects.
- Brush up on polymorphism syntax and be prepared to write code examples on the spot.
- Have practical examples of polymorphism in Java, such as comparing different types of collections.
- Familiarize yourself with the different design patterns and be prepared to explain how they relate to polymorphism.
- Understand the pros and cons of using polymorphism, such as when it is more advantageous than using inheritance.
- Be familiar with the different types of polymorphism, such as parameterized, generic, and object- oriented polymorphism.
- Understand the concept of polymorphic class hierarchies and be able to explain why they are useful.
- Be able to differentiate between static and dynamic polymorphism.
- Be prepared to explain how to create a polymorphic method in Java and how to call it from other methods.
- Be able to write code to demonstrate the use of polymorphism in a program.
- Understand the differences between method overloading and method overriding.
- Be familiar with the concept of generics and how they can be used in Java polymorphism.
- Be able to explain the different levels of polymorphism and how they are used in Java.
- Understand how interfaces are used to implement polymorphism in Java and have examples of how they can be used.
Conclusion
Java polymorphism is a powerful tool for developing object- oriented applications. It allows for the creation of reusable code and object hierarchies that can easily be maintained and extended. By understanding the fundamentals of polymorphism and the corresponding interview questions, Java developers can demonstrate their knowledge of the language to potential employers. With the right guidance and practice, developers can use this knowledge to boost their career and develop more robust software solutions.