Design patterns have become an integral part of the software engineering process. With the introduction of object-oriented programming, design patterns have been used to help developers create applications that are more maintainable and scalable. Design patterns allow developers to reuse code, making their applications more efficient and cost effective.
Design patterns are great for software engineers looking for a framework to build applications on. C# is a popular language for developing software applications and design patterns are a necessary tool for developing efficient and maintainable applications. It is important for software engineers to understand the different design patterns and how to implement them in C#.
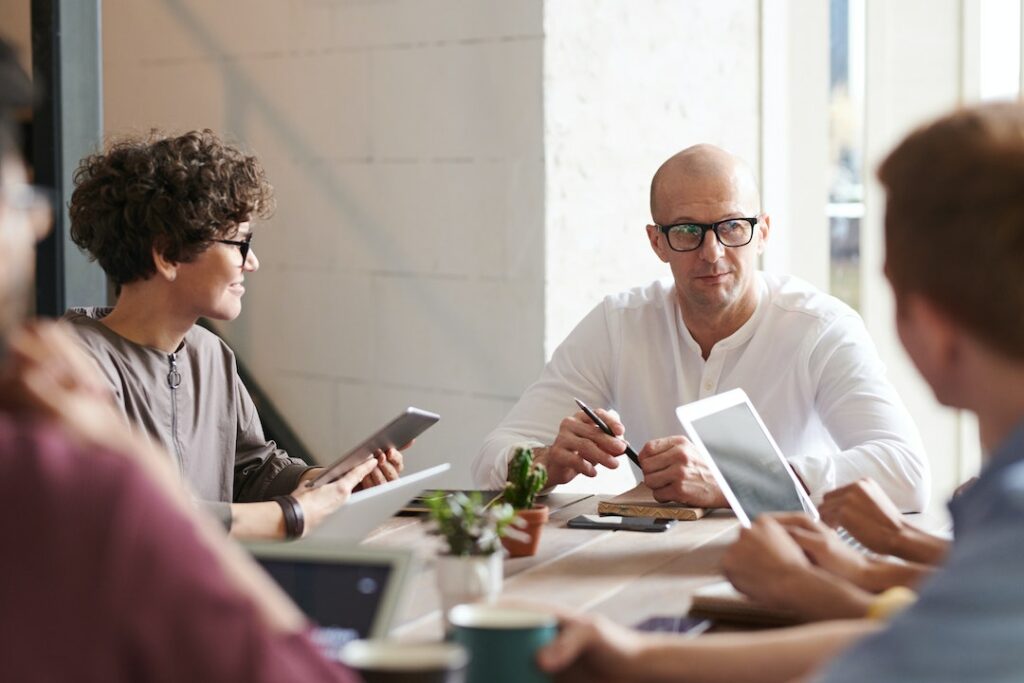
The following blog post provides some of the most commonly asked questions and answers about design patterns in C# interviews. It is a great resource for software engineers who want to increase their knowledge about design patterns and gain a better understanding of how to use them in C# applications. The blog post covers topics such as the Singleton pattern, the Factory pattern, the Strategy pattern, the Observer pattern, and more. It also provides sample code snippets to illustrate how to use these patterns in a C# application.
By reading this blog post, software engineers can gain a better understanding of the basics of design patterns and how to apply them in their C# projects. This blog post is a great resource for software engineers who are looking to brush up on their skills before their next C# interview.
Overview of Design Patterns C# Interview Process
Design patterns are an important part of the C# interview process. They are used to identify a programming skill set and the candidate’s ability to work with the language. Design patterns are reusable solutions to commonly occurring software engineering problems. They can provide a consistent structure to a system, allowing for more efficient and effective development.
The C# interview process usually starts with a general discussion of the candidate’s experience in coding and software engineering, as well as their knowledge of design patterns. This part of the interview is usually conducted in the form of a Q&A session. The interviewer will then ask the candidate to explain and demonstrate the design patterns they are familiar with. For each pattern, the candidate should be prepared to describe how it works and how it is used in software engineering.
The interviewer may also ask the candidate to provide a code example of a design pattern they are familiar with. The code should demonstrate an efficient and effective use of the pattern. The interviewer will be looking to assess the candidate’s knowledge of the pattern, as well as their ability to write clear and concise code.
The final part of the C# interview process usually involves a discussion of the candidate’s experience with the language. This can include questions about their experience using the .NET framework and any other development tools. The interviewer may also ask about the candidate’s experience debugging and optimizing code. The interviewer will be looking to assess the candidate’s ability to work with the language and their understanding of the fundamentals of software engineering.
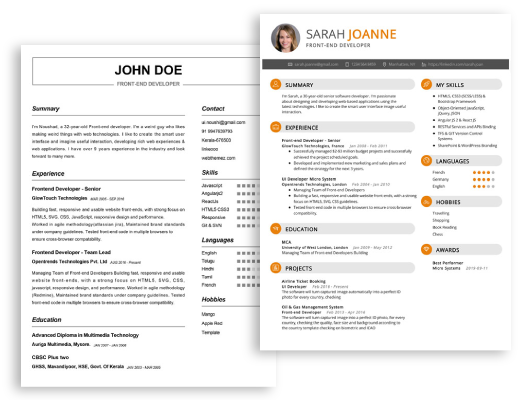
Start building your dream career today!
Create your professional resume in just 5 minutes with our easy-to-use resume builder!
Be sure to check out our resume examples, resume templates, resume formats, cover letter examples, job description, and career advice pages for more helpful tips and advice.
Top 15 Design Patterns C# Interview Questions and Answers
1. What is a design pattern?
Design patterns are reusable solutions to common problems in software design. They provide a way of thinking about a problem and its potential solutions, allowing developers to quickly identify and implement the most effective solution. Design patterns are language-independent, and can be implemented in any language, including C#.
2. What are the different types of design patterns?
There are three main types of design patterns: creational, structural, and behavioral. Creational patterns provide ways to create objects and organize classes and objects. Structural patterns focus on how classes and objects can be organized to form larger structures or subsystems. Behavioral patterns focus on how classes and objects interact and communicate with each other.
3. What is the Singleton design pattern?
The Singleton design pattern is a creational pattern which restricts the instantiation of a class to one instance. This is useful when only one instance of a class is required, for example when managing a shared resource. The Singleton pattern ensures that only one instance of the class is created, and provides a global access point to that single instance.
4. What is the Prototype design pattern?
The Prototype pattern is a creational pattern which allows an object to be cloned or copied when creating new instances of that object. This is useful when creating objects that are expensive to construct, such as complex objects with many fields or complex calculations. By using the Prototype pattern, the expensive construction process can be avoided and a clone of an existing object can be created instead.
5. What is the Adapter design pattern?
The Adapter design pattern is a structural pattern which allows two incompatible interfaces to work together. This is achieved by creating a wrapper class which implements the target interface and which delegates the calls to the existing incompatible class. This allows the existing class to be used in situations where it would otherwise be incompatible.
6. What is the Facade design pattern?
The Facade design pattern is a structural pattern which provides a simplified interface for a complex system. It wraps a complicated subsystem with a simpler interface, allowing clients to interact with the subsystem without needing to understand its complexity. This simplifies the usage of the subsystem, allowing the client code to be simpler and more maintainable.
7. What is the Builder design pattern?
The Builder design pattern is a creational pattern which separates the construction of complex objects from its representation. This is achieved by using a builder object which knows how to construct the object, and a director object which knows what steps are required to create the object. This allows the construction process to be decoupled from the representation of the object, simplifying the code and making it easier to maintain.
8. What is the MVC design pattern?
The Model-View-Controller (MVC) design pattern is a structural pattern which provides a way of separating an application into three distinct layers: the model, which represents the data of the application; the view, which is responsible for displaying the data to the user; and the controller, which controls the flow of the application. This provides separation of concerns, making the code easier to maintain and allowing for easier unit testing.
9. What is the Observer design pattern?
The Observer design pattern is a behavioral pattern which allows objects to be notified of events occurring in other objects. This is achieved by having a ‘subject’ object which notifies ‘observer’ objects of changes, allowing them to be kept up to date with the state of the subject. This is useful for applications that require data to be synchronized across multiple objects.
10. What is the Strategy design pattern?
The Strategy design pattern is a behavioral pattern which allows algorithms to be swapped in and out at runtime. This is achieved by creating a strategy interface which defines a specific algorithm, and creating concrete classes which each implement the interface. This allows the algorithm to be swapped out as needed, without needing to modify the code that uses the algorithm.
11. What is the Command design pattern?
The Command design pattern is a behavioral pattern which allows operations to be encapsulated in objects. This is achieved by creating a command interface which defines an execute method, and creating concrete classes which implement the interface. This allows operations to be passed around, queued, and undone as needed.
12. What is the Mediator design pattern?
The Mediator design pattern is a behavioral pattern which allows objects to communicate with each other without needing to know about each other. This is achieved by creating a mediator object which handles communication between the objects, allowing them to maintain loose coupling and be independent of each other. This simplifies the communication between objects, making the code easier to maintain.
13. What is the Template Method design pattern?
The Template Method design pattern is a behavioral pattern which allows the implementation of an algorithm to be shared by multiple classes. This is achieved by defining a template method which contains the steps of the algorithm, and then allowing subclasses to override specific steps of the algorithm as needed. This allows the algorithm to be easily reused and extended.
14. What is the Interpreter design pattern?
The Interpreter design pattern is a behavioral pattern which allows a language to be interpreted. This is achieved by creating an expression tree, which contains objects which represent parts of the language. This expression tree can then be evaluated to interpret the language and produce the desired result.
15. What is the Flyweight design pattern?
The Flyweight design pattern is a structural pattern which allows multiple objects to share data. This is achieved by creating a flyweight object which contains the data, and then using multiple objects which reference the flyweight object. This allows objects to be created quickly, saving memory and improving performance.
Tips on Preparing for a Design Patterns C# Interview
- Research the SOLID principles of object- oriented programming principles as these are key to understanding design patterns in C#.
- Become familiar with the different types of design patterns, such as the Singleton, Factory, and Observer patterns.
- Understand how the different types of design patterns are used in C# and the advantages and disadvantages of each.
- Read up on the various refactorings associated with design patterns, such as the Open/Close Principle and Dependency Inversion Principle.
- Become familiar with the different design patterns implementations in the .NET framework, such as the Iterator, Composite, and Facade patterns.
- Practice coding examples with the various design patterns in C#.
- Have a few code samples ready to share during the interview.
- Be prepared to explain the purpose and various use cases of a given design pattern, if asked.
- Understand the importance of using design patterns to improve software design and how they can help reduce the complexity of an application.
- Be able to explain why a particular design pattern was chosen for a given situation.
- Be prepared to answer questions about the underlying principles and techniques used in the design patterns.
- Understand the impact of design patterns on performance, scalability, and maintainability.
- Be able to explain how different design patterns can be combined to create a solution.
- Understand the different architectures and patterns used in C# development, such as Model- View- Controller (MVC) and Model- View- ViewModel (MVVM).
- Have a good understanding of the differences between procedural, object- oriented, and functional programming.
Conclusion
The post on Design Patterns C# Interview Questions and Answers was a comprehensive overview of the most important topics to consider when preparing for an interview. It provided a variety of questions and answers that can be used to test a candidate’s knowledge on the topic. By understanding the fundamentals of design patterns and getting a good grasp of the different types of C# questions, you can increase your chances of success in an interview. With this knowledge in hand, you can now go into your next interview with more confidence and better prepared to answer the questions that may come your way.