Swift is a programming language developed by Apple in 2014 that has quickly become one of the most popular languages for developing mobile applications. With the increasing demand for mobile applications, job opportunities for Swift developers are on the rise. As a result, job seekers should prepare for Swift-related interview questions.
In this blog post, we’ll provide a comprehensive guide to Swift interview questions and answers. We’ll cover topics such as the basics of Swift, code structure, syntax, memory management, and Apple’s development tools. With our tips and sample answers, you’ll be prepared to tackle any Swift-related question thrown your way in an interview.
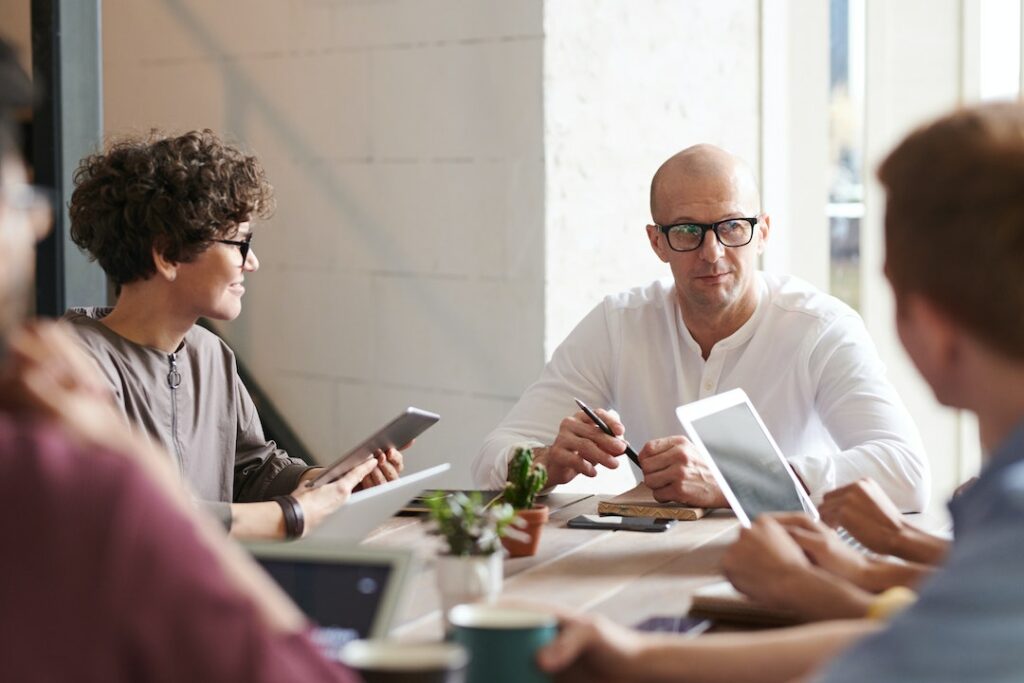
Whether you’re a seasoned Swift developer or just starting out, this blog post has something for everyone. We’ll start off with a brief overview of the language, then move on to the more advanced topics. If you’re looking for a refresher on the basics, we’ll cover the fundamentals of Swift, including syntax, code structure, and memory management. We’ll also provide tips to make sure your answers stand out from the rest.
So, if you’re ready to prepare for your next Swift interview, let’s get started! From the basics to the more advanced topics, this blog post will provide you with all the Swift interview questions and answers you need to ace your interview.
Overview of Swift Interview Process
The Swift interview process begins with the initial phone screen between the candidate and the hiring manager. This is a chance for both parties to get to know one another, discuss the role and team, and understand the candidate’s skills and qualifications. Following the phone screen, a technical interview is typically conducted. This interview tests the candidate’s technical knowledge, problem- solving abilities, and coding experience. During this stage, the interviewer will ask the candidate a series of questions to assess their skill set and determine if they are a good fit for the team.
The third stage of the Swift interview process is the on- site interview. This is the most comprehensive step of the process and will typically involve multiple interviews with various team members. The team will ask a series of technical questions that are related to the role and job requirements, as well as questions about the candidate’s experience and background in the field. The hiring team will also assess the candidate’s communication and collaboration skills, as well as their ability to work in a team environment.
After the on- site interview, the hiring team will review the candidate’s responses and evaluate their overall performance. If they feel the candidate is a good fit for the role, they will make an offer. If the candidate accepts, they will be given an official start date and be welcomed to the team.
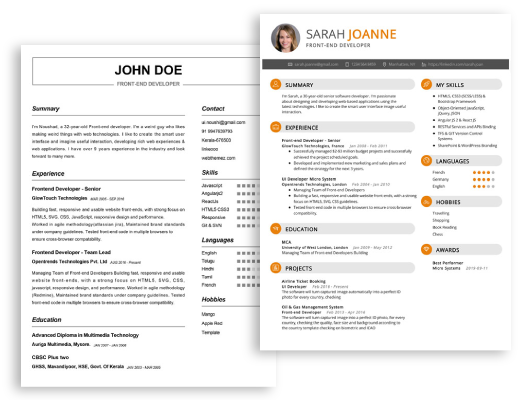
Start building your dream career today!
Create your professional resume in just 5 minutes with our easy-to-use resume builder!
Be sure to check out our resume examples, resume templates, resume formats, cover letter examples, job description, and career advice pages for more helpful tips and advice.
Top 17 Swift Interview Questions and Answers
1. What is Swift?
Swift is a powerful and intuitive programming language for macOS, iOS, watchOS, tvOS, and beyond. It was developed by Apple in 2014 as the successor to their Objective-C language. Swift is designed to be easy to learn and use, with a concise, modern syntax and powerful features like generics, closures, and type inference. It is designed to be both safe and fast, with built-in support for memory management, type safety, and automatic reference counting (ARC). Swift is also fully interoperable with Objective-C, allowing developers to use existing Objective-C libraries while taking advantage of the modern features available in Swift.
2. What are the advantages of using Swift?
The advantages of using Swift include improved readability and speed of development, as well as the ability to easily maintain and debug code. Swift’s syntax is designed to be less verbose than Objective-C, making it easier to read and write. It also includes powerful features like generics, closures, and type inference that allow developers to write less code and get more done. Additionally, Swift is highly optimized for modern hardware, so apps written in Swift run faster and more efficiently.
3. What is the difference between Swift and Objective-C?
The main difference between Swift and Objective-C is that Swift is a modern, type-safe language designed for improved readability and speed of development, while Objective-C is an older, non-type-safe language designed for compatibility with C. Additionally, Swift eliminates the need for header files and has built-in support for memory management, type safety, and automatic reference counting (ARC).
4. Why is Swift a better choice than Objective-C?
Swift is a better choice than Objective-C for several reasons. First, Swift is a modern, type-safe language designed to be easier to read and write than Objective-C. Second, Swift includes powerful features like generics, closures, and type inference that allow developers to write less code and get more done. Finally, Swift is highly optimized for modern hardware, so apps written in Swift run faster and more efficiently.
5. What is Swift?
Swift is a modern programming language developed by Apple to create applications for iOS, macOS, watchOS, tvOS and more. It was designed to replace the Objective-C language and make development easier, faster and safer. Swift is an intuitive, open source and powerful language that is easy to learn and use. It is a type-safe language, which means that it ensures type safety during compile time, preventing errors and improving readability of code.
6. What are the advantages of using Swift?
The main advantages of using Swift are its readability, safety and speed. Swift code is easier to read and write than most other programming languages, and its type safety ensures fewer errors due to incorrect data types. Swift is also much faster than Objective-C, making it a great choice for developing apps for Apple products. Additionally, Swift is an open source language, so developers can access its source code and modify it as needed.
7. What is the difference between Swift and Objective-C?
The biggest difference between Swift and Objective-C is that Swift is a type-safe language while Objective-C is not. This means that Swift code is checked for type errors during compile time, while Objective-C code is not. Swift is also much faster than Objective-C, making it a better choice for developing applications for Apple products. Additionally, Swift is an open source language, so developers can access its source code and modify it as needed.
8. What is an Optional in Swift?
An Optional in Swift is a type that can either contain a value or nil. Optionals are used to handle situations where a variable may or may not have a value. For example, an optional string might contain a value like “hello” or nil if it is empty. Optionals help to prevent runtime errors by allowing us to check for a value before using it.
9. What is the difference between let and var in Swift?
The main difference between let and var in Swift is that let is used to declare a constant and var is used to declare a variable. Constants declared with the let keyword cannot be changed, while variables declared with the var keyword can be changed. Constants are often used when you know a value won’t change, while variables are used when you want a value to be able to change.
10. What is a Struct in Swift?
A Struct in Swift is an easily accessible type, which consists of related values and can be used to store data, define methods and create custom types. Structs are commonly used to represent data that is more complex than a primitive type such as a string or number, and they are also useful for defining custom types.
11. What is a Protocol in Swift?
A Protocol in Swift is a type that defines the properties and methods that a conforming type must implement. Protocols can be used to define a common interface for different types that can then be used together. For example, a Protocol can be used to define a method for data serialization, and then any type that conforms to that Protocol can use that method.
12. What are the main differences between classes and structs in Swift?
The main difference between classes and structs in Swift is that classes can inherit from other classes and structs cannot. Additionally, structs are value types, meaning that when you assign a struct to a variable or pass it to a method, a copy of the struct is created. Classes are reference types, which means that when you assign a class to a variable or pass it to a method, only a reference to the class is created.
13. What is the difference between functions and closures in Swift?
The main difference between functions and closures in Swift is that functions are named and can be stored in a variable, while closures are anonymous and cannot be stored in a variable. Additionally, closures can capture and store references to any constants and variables from the context in which they are defined. This allows for greater flexibility when writing complex code with functions and closures.
14. How does Swift handle memory management?
Swift uses Automatic Reference Counting (ARC) to manage memory. ARC automatically keeps track of the objects that an application is using and when they are no longer needed, it frees up the memory for other objects. ARC also ensures that objects that are still being used are not released from memory. This makes it easier to manage memory in applications and ensures that memory leaks are not a problem.
15. What are protocols in Swift?
Protocols in Swift are a way to define a common set of requirements for classes and other types. Protocols define the methods and properties that must be implemented in order for a type to conform to a given protocol. Protocols are declared using the protocol keyword, and are typically used when writing frameworks and reusable libraries.
16. What is the difference between a struct and a class in Swift?
The main difference between a struct and a class in Swift is that structs are value types, while classes are reference types. Structs are stored in memory in their entirety, while classes are stored in memory as references to their data. Additionally, structs are generally used for simpler data types, while classes are used for more complex data types.
17. What are generics in Swift?
Generics in Swift are a way to write code that can work with a variety of types. Generics allow developers to write code that is generic, meaning it can work with any type instead of being tied to a specific type. This allows developers to write more flexible and reusable code. Generics are declared using the < and > symbols, and are typically used when writing frameworks and reusable libraries.
Tips on Preparing for a Swift Interview
- Research the company: Make sure you understand the company’s products, mission, and values.
- Understand Swift: Make sure you are familiar with the language and its core concepts, including classes, protocols, generics, and memory management.
- Practice coding: Try to solve coding challenges or practice programming in Swift.
- Review your work: Look back at the projects you’ve completed in Swift and prepare to articulate them.
- Create an example project: Build a small project in Swift that showcases your skills.
- Prepare your portfolio: Have a portfolio of your best work ready to show potential employers.
- Review your resume: Make sure your resume reflects your skills, relevant experience, and accomplishments in Swift.
- Practice your answers: Prepare for the questions you might be asked and practice your responses.
- Bring a copy of your code: Have a copy of your code ready in case you need to show it during the interview.
- Dress appropriately: Make sure you dress in smart, professional clothing that is appropriate for the company.
- Be confident: It is important to exude confidence during the interview.
- Be prepared to answer questions: Be prepared to answer questions in a timely manner and to explain your thought process.
- Be proactive: Show enthusiasm and interest in the role and be willing to ask questions.
- Follow up: Send a thank you note after the interview to reiterate your interest in the position.
- Stay positive: Keep a positive attitude and remain confident in your skills and experience.
Conclusion
Swift is a powerful and versatile programming language used to develop apps for macOS, iOS, watchOS, and tvOS. Knowing how to answer the most common job interview questions about Swift can help you stand out from the other applicants and increase your chances of getting the job. In this blog, we have compiled some of the most commonly asked questions about Swift along with their answers. We hope this guide will be helpful for you to prepare for your next Swift interview and help you secure your dream job. Good luck!