Java is one of the most popular programming languages used by software developers and it is highly sought after by employers. As such, employers often use Java interviews as part of their recruitment process. Java interview questions are designed to assess a candidate’s knowledge of the language and their ability to apply it in a practical setting.
Answering these questions can be tricky, as they require a deep understanding of Java and its capabilities. Therefore, it is important to prepare for a Java interview by familiarizing yourself with the topics that are commonly asked. This blog post will provide a comprehensive list of the most common Java interview questions and their answers.
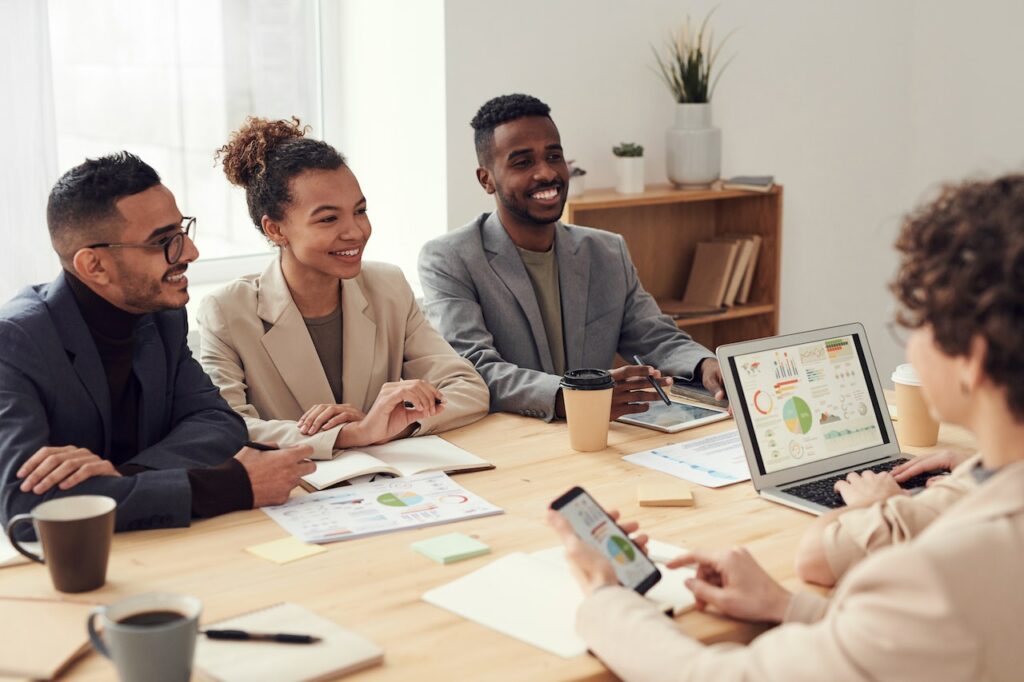
We will cover topics such as object-oriented programming, collections and generics, threading and synchronization, exception handling, and the Java language fundamentals. We will also provide tips on how to approach each question, as well as advice on how to ace the Java interview.
By the end of this blog post, you will have a clear understanding of the most common Java interview questions and how to answer them. With this knowledge, you will be able to confidently present yourself to any Java interview and demonstrate your skills.
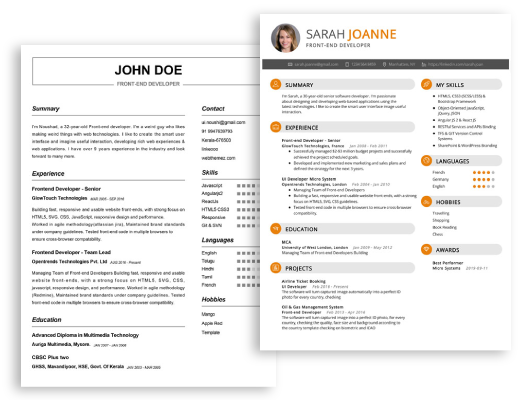
Start building your dream career today!
Create your professional resume in just 5 minutes with our easy-to-use resume builder!
Overview of Java Interview Process
The Java interview process is typically very thorough and can involve a wide range of different activities. Generally speaking, the process starts with a telephone or video interview to determine if the candidate is a good fit for the position. During this initial interview, the recruiter or hiring manager will ask questions about the candidate’s background, experience with Java, and general knowledge of software development. If the candidate passes this initial phase, they will typically be invited to an in- person interview, where a more in- depth technical assessment may be conducted.
During the in- person interview, the interviewer or panel may ask questions about the candidate’s experience with Java and its related technologies. Candidates may also be asked to complete coding challenges or solve problems using Java. At this stage, the interviewer may also ask the candidate to explain their design choices and explain the code they have written. Depending on the position, the interviewer may also ask questions about the candidate’s knowledge of software engineering best practices, such as design patterns, unit testing, and source control.
If a candidate passes the in- person interview, they may be asked to complete a take- home coding challenge. This challenge will usually focus on solving a specific problem using Java and related technologies. After the candidate has submitted their solution, the interviewer will evaluate the candidate’s code and may ask them to explain their decisions. The interviewer may also ask the candidate to make any necessary improvements to the code.
Finally, if the candidate passes all the stages of the interview process, they may be offered the job. The hiring manager or recruiter may then ask the candidate to provide references or discuss the details of the job offer, such as the salary and start date. After the offer has been accepted, the candidate may also need to complete a background check and drug test before they can begin working.
Top 20 Java Interview Questions and Answers
Be sure to check out our resume examples, resume templates, resume formats, cover letter examples, job description, and career advice pages for more helpful tips and advice.
1. What is Java?
Java is an object-oriented, general-purpose programming language created by Sun Microsystems that enables developers to create robust, secure, and dynamic applications that can run across multiple platforms. Java is used to develop a wide range of applications, from simple desktop applications to complex web applications. Java is also a popular language for mobile devices, allowing developers to create applications for Android and other mobile operating systems.
2. What are the main features of Java?
The main features of Java include:
- Object-oriented: Java is an object-oriented programming language, meaning that all of its operations are conducted through objects. This allows for modular programming, making it easier to debug and maintain code.
- Platform independent: Java programs can be executed on any platform, making it a popular choice for web development.
- High performance: Java is an interpreted language, which means it is compiled at runtime rather than pre-compiled. This means that Java can execute code faster than other languages.
- Secure: Java is designed with security in mind, and has many built-in security features that make it difficult for malicious code to be executed.
3. What is a Java Virtual Machine?
A Java Virtual Machine (JVM) is a software implementation of a virtual machine that enables a computer to run Java programs. JVMs are platform-independent and can be used on any operating system. The JVM is responsible for running Java bytecode, which is the compiled form of Java source code. It also provides a framework for the implementation of Java classes and objects, as well as other features such as garbage collection.
4. What is an Interface in Java?
An interface in Java is a collection of abstract methods and constants that must be implemented by any class that implements the interface. An interface serves as a contract between different classes, specifying which methods and constants must be present in order for the classes to be considered compatible. Interfaces can also be extended to create a hierarchy of related interfaces.
5. What is garbage collection in Java?
Garbage collection in Java is the process of reclaiming memory that is no longer being used by an application. The Java Virtual Machine (JVM) uses a garbage collector to identify objects in memory that are no longer needed, and reclaims the memory occupied by those objects. This allows applications to use resources efficiently, as the JVM can free up memory when it is no longer needed.
6. What is a constructor in Java?
A constructor in Java is a special method that is used to initialize an object when it is created. Constructors are typically used to set initial values for an object’s instance variables. Constructors must have the same name as the class and do not have a return type.
7. What is a Java package?
A Java package is a collection of related classes and interfaces that are bundled together in order to facilitate code reuse and organization. Packages are used to organize related classes and interfaces, and can be imported into other classes to give them access to the classes and interfaces within the package.
8. What is the difference between an interface and an abstract class?
An interface is a collection of abstract methods and constants that must be implemented by any class that implements the interface. An abstract class is a class that cannot be instantiated, and contains both abstract methods and concrete methods. Unlike interfaces, abstract classes can define fields and implement methods, as well as define constructor methods.
9. What is the purpose of the main method in Java?
The main method in Java is the entry point of any Java application. The main method is the method that is executed when the program is run. The main method is typically the first method in the program, and is used to launch the application.
10. What is the difference between JDK, JRE, and JVM?
The Java Development Kit (JDK) is a bundle of software development tools that includes the Java Runtime Environment (JRE) and the Java Virtual Machine (JVM). The JRE is the environment in which Java programs are executed, and the JVM is the process responsible for running Java bytecode. The JDK provides the tools necessary to compile, debug, and execute Java programs.
11. What is the most important feature of Java?
The most important feature of Java is its platform independence. Java was designed to be platform independent and is able to run on any hardware or operating system without any modifications. Java also has a robust set of features that make it a powerful language for building software applications, including an object-oriented programming model, a large standard library of packages, and an intuitive syntax. Additionally, Java is designed to be secure and maintainable, with built-in support for memory management, exception handling, and stringent security protocols.
12. What is the difference between JDK, JRE, and JVM?
Java Development Kit (JDK) is a software package that contains the necessary tools and libraries for developing Java applications. It includes a Java Runtime Environment (JRE) and a Java virtual machine (JVM), which are essential components for running Java applications. The JRE is the runtime environment that enables running Java programs, while the JVM is an implementation of the JRE that is specific to a particular platform or operating system. In addition to the JVM, the JDK includes a compiler, an interpreter, debugging tools, and other development tools.
13. What is the purpose of garbage collection in Java?
Garbage collection is the process of reclaiming memory occupied by objects that are no longer in use. It is an important feature of Java, as it helps reduce memory leaks and other issues related to memory management. Garbage collection is performed automatically by the JVM, and it works by periodically scanning the memory for objects that are no longer referenced by any running programs. Once these objects are identified, the JVM reclaims the memory occupied by them.
14. What is the difference between an interface and an abstract class?
An interface is a group of related methods with empty bodies, which a class can implement. An abstract class is a class with one or more abstract methods, which must be implemented by any class that extends the abstract class. Interfaces provide a way to define a contract between different classes, while abstract classes define the structure of a class and provide a base from which to extend. Interfaces can only contain abstract methods, while abstract classes can contain both abstract and concrete methods.
15. What do you mean by immutable objects?
Immutable objects are objects whose state cannot be modified once they are created. These objects are guaranteed to maintain their state throughout their lifetime, which makes them thread-safe and ideal for use in multi-threaded applications. Examples of immutable objects in Java include String, Integer, and Boolean.
16. What is the difference between a constructor and a method?
A constructor is a special type of method used to create new objects. It has the same name as the class, and it is automatically called when an object is created. A constructor does not have a return type, and it is used to initialize the attributes of the object. A method, on the other hand, is a subroutine that performs a specific task. A method can be declared with a return type, and it can take parameters as arguments.
17. What is the purpose of the static keyword in Java?
The static keyword is used to refer to a class member that can be accessed without creating an instance of the class. It is used for methods and variables that are shared by all instances of a class. Static variables are stored in the class area, and they are initialized when the class is loaded. Static methods can be invoked without creating an instance of the class, and they can only access static variables.
18. What is the difference between a String and a StringBuffer in Java?
A String is an immutable sequence of characters, which means its value cannot be changed once it has been created. A StringBuffer is a mutable sequence of characters, which means that its value can be changed after it has been created. A StringBuffer is more efficient than a String for performing a series of operations, as it can be modified without creating a new object each time.
19. What is the difference between an Array and an ArrayList in Java?
An array is a static data structure that stores a fixed-size collection of elements of the same type. An ArrayList is a dynamic data structure that stores an expandable collection of elements of the same type. An array is more efficient than an ArrayList for performing operations on a fixed size of elements, as it stores the elements in contiguous memory locations.
20. What is the purpose of the final keyword in Java?
The final keyword is used to indicate that a variable or method cannot be changed. It is used to prevent a variable from being reassigned and to prevent a method from being overridden. The final keyword can also be used to indicate that a class cannot be extended, which is useful for preventing inheritance-related issues.
Important Tips for Preparing Java Interview
- Familiarize yourself with the most commonly used Java APIs and libraries.
- Review the basics of object- oriented programming and the fundamentals of the Java language.
- Prepare examples of your work with Java to demonstrate your programming experience.
- Practice technical questions and interview puzzles related to the Java language.
- Know the fundamentals of algorithms and data structures and how they are used in Java.
- Rehearse your answers to potential interview questions to help reduce pre- interview anxiety.
- Research the company and be prepared to discuss how your skills and experience would be a good fit.
- Develop a portfolio of relevant projects you’ve done that use Java.
- Be prepared to discuss your experience with debugging and troubleshooting Java code.
- Know the different Java frameworks and technologies used in the industry.
- Have a basic understanding of database systems and how they interact with Java.
- Understand the various design patterns used in Java development.
- Be prepared to discuss your experience with writing unit tests.
- Be able to explain the unique features of the Java language and its advantages over other languages.
- Be prepared to discuss how you would design a Java application from start to finish.
Conclusion
In conclusion, the Java Interview Questions and Answers blog has been a great resource for aspiring Java developers. It has provided a comprehensive list of questions and answers that can help candidates prepare for their interviews. It has also offered a valuable insight into the core concepts and principles of the language. With the help of this blog, candidates can feel confident that they have the knowledge and skills needed to ace their Java interviews.